In todays post we will see how to download Instagram image using C#.
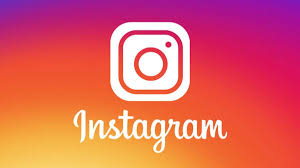
Instagram is the most popular social media platform that emphasizes photo and video sharing. It allows you to take, edit and publish visual content for your followers. Unlike Facebook, Instagram’s sole purpose is to enable users to share images or videos with their audience.
Having said that, Instagram makes it hard to download photos to your computer. But it is possible. And this tutorial will guide you through everything you need to know on how to build a C# application that can download your favorite Instagram post.
This lesson is for educational purposes only. If you are downloading Instagram images, please respect image ownership. And always seek permission and give credit where credit is due.
Although there are many ways to download Instagram post, we will retrieve it by a procedure called scraping. Web scraping is a process used for extracting data from web sites. This C# application will be able to access the Instagram photo directly using the Hyper Text Transfer Protocol. There will be a complete section in this tutorial on how to use C# to load the Instagram post URL and render the entire web site. As a result, we will be able to locate and scrape the desired image.
How to use the Instagram Photo Download App
For demonstration purposes we will download a Peter McKinnon photo.
Peter McKinnon is a Canadian YouTuber, photographer, filmmaker, and vlogger. He is most known for his filmmaking and photography tutorials mainly using Adobe Premiere Pro and Adobe Lightroom.
Today we will scrape one of his amazing posts. So, lets find an image that we like.
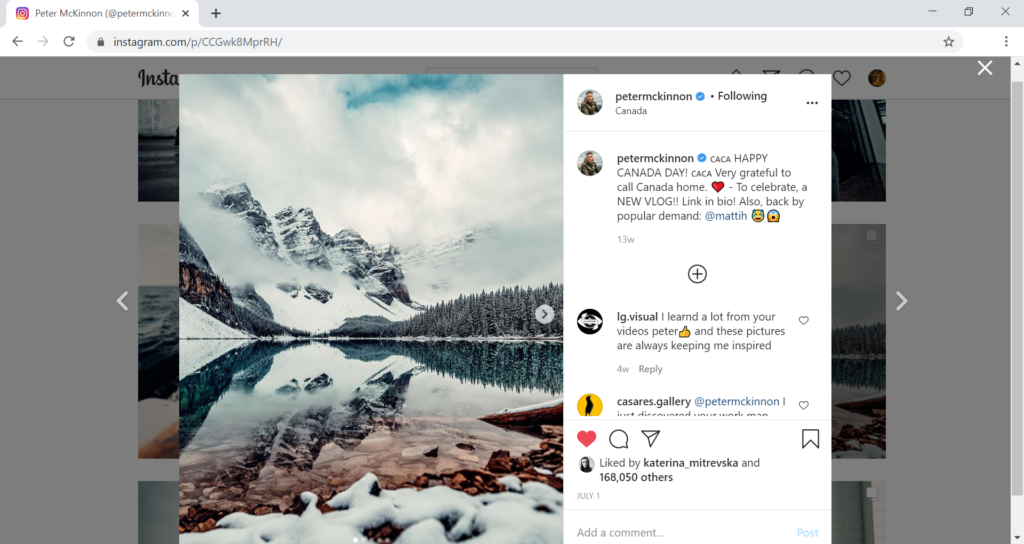
Then open it and copy the photo URL address in the C# application.
https://www.instagram.com/p/CCGwk8MprRH/
Next, choose your download location, and click download. It is as simple as that.
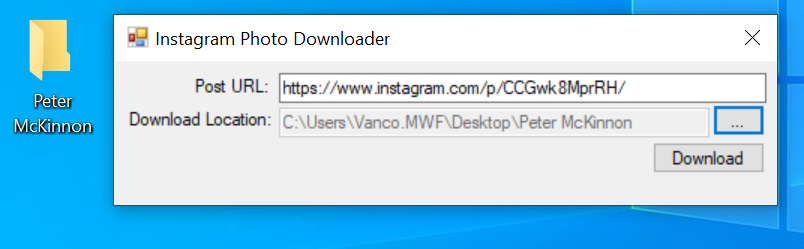

How to scrape Instagram Post with C#
As we mentioned earlier, first we need to download the web page source code. For that we will be using the Web Client class. Open and establish a connection with the URL site and download the source code as string.
public async Task<string> DownloadPostSourceCode(Uri webPageUri)
{
using (WebClient client = new WebClient())
{
return await client.DownloadStringTaskAsync(webPageUri);
}
}
Inside the web page source code there is a URL location pointing to the image we want to download. Finding the image address is not that easy. There is a lot of text to search through. But there is pattern to how the destination string is constructed. Let us find it.
"((""display_url"":""https://).*?(p1080x1080)?(oe=[A-Z0-9]{5,}""))"
This text represents a regular expression. A regular expression is a pattern that the regular expression engine attempts to match in input text.
A pattern consists of one or more-character literals, operators, or constructs. This C# code is searching for a text that starts with:
“display_url”:”https:// then it is followed by the p1080x1080 string and ends with oe={uppercase letter/number combination}”
But before that we need to un-escape the string. Inside the downloaded web page source, the image URL contains escaped & “ampersand” character.
To unescape it using C# syntax, we can simply use: Regex.Unescape(string)
Once we have obtained the post URL we can extract the image file name with the following expression:
"[0-9]*_[0-9]*_[0-9]*_n.jpg"
Next, for every string matched by our regular expression engine, we can download the photo using the Web Client class again. But, this time we will be opening a readable stream for the data downloaded from a resource with the URL specified as a parameter.
using (WebClient webClient = new WebClient())
{
using (Stream stream = webClient.OpenRead(imageUri))
{
return Image.FromStream(stream);
}
}
As a result, we can create an Image instance from the provided stream.
All we have to do now is save the image on our hard drive
image.Save({image_location});
Download Instagram Image with C# source code
The source code can be downloaded from the following link