In this article we are going to learn how to parse RSS and ATOM Feeds in C#. The source code is provided at the end of the article.
This small project will be a part of a content aggregator that we are going to build. This first step will focus on creating an RSS feed parser. This way we can get updates from our favorite web sites.
What is an RSS feed?
RSS is a web feed that allows users and applications to access updates to websites in a standardized, computer-readable format. This app will allow us to keep track of many different web sites in a single content aggregator. Therefore, this aggregator will check the RSS feed for new content on a daily basis and classify it with regards to the user preferences.
How does RSS works?
RSS stands for Really Simple Syndication. It refers to files easily read by a computer called XML files that automatically update information.
This information is fetched by a user’s RSS feed reader. Then it converts the files into the latest updates from websites. It feeds you headlines, summaries, update notices and links back to articles on your favorite website page.
Knowing this we are going to use RSS to get updates from our favorite websites and we will create a ML project that will display only the articles that might interest us.
How to Parse RSS and ATOM Feeds
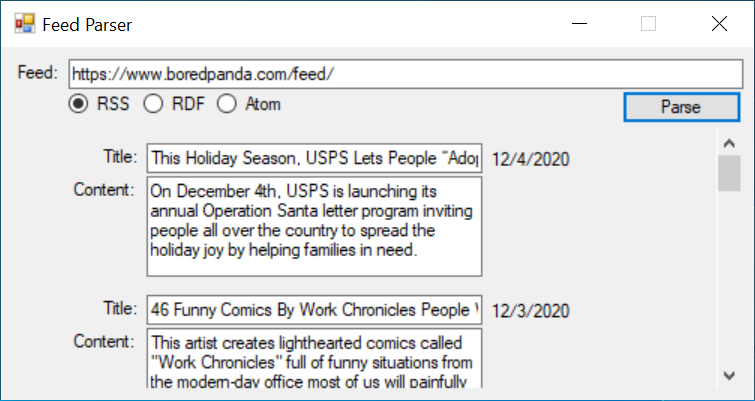
As you can see where are going to build an application that is capable of parsing RSS, RDF and Atom feeds.
One way to parse the feed is to read the feed from a URL into XDocument:
XDocument doc = XDocument.Load(feedUrl.Url);
Like we mentioned. RSS is nothing more then a XML document so we can easily use XDocument class. Also we can use LINQ to query the document structure.
There are some differences between the RSS, RDF and Atom feeds in the document structure.
Query RSS Feed
To Query RSS feed we need to have in mind the following structure:
RSS/Channel/item
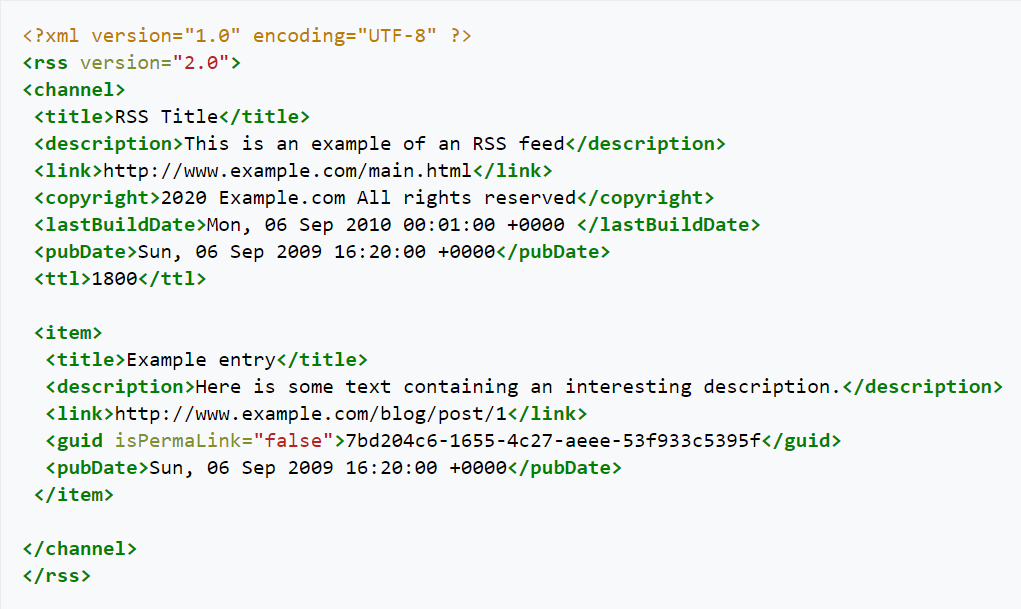
Knowing that we can finally query our document like so:
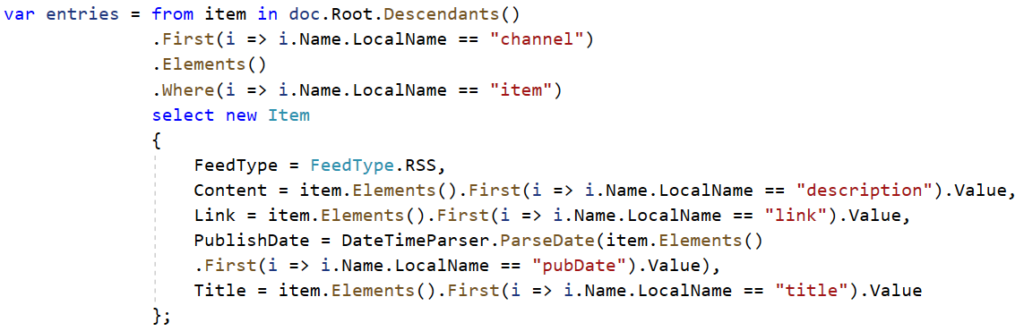
Query RDF Feed
To Query RDF feed we need to have in mind the following structure:
<item> is under the root
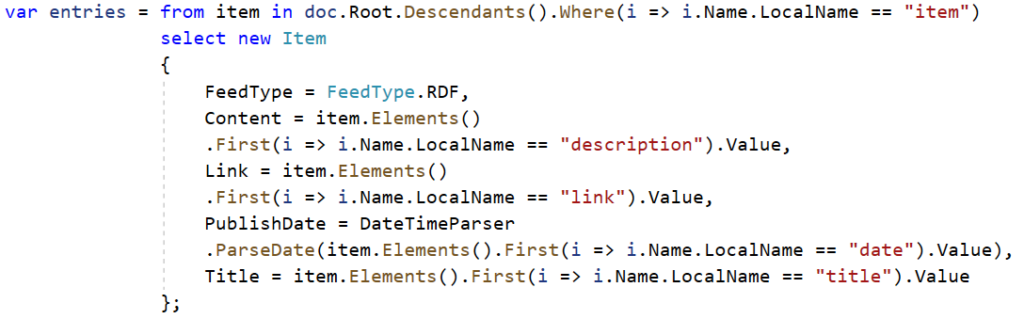
Query Atom Feed
To Query Atom feed we need to have in mind the following structure:
Feed/Entry

Knowing that we can finally query our document like so:
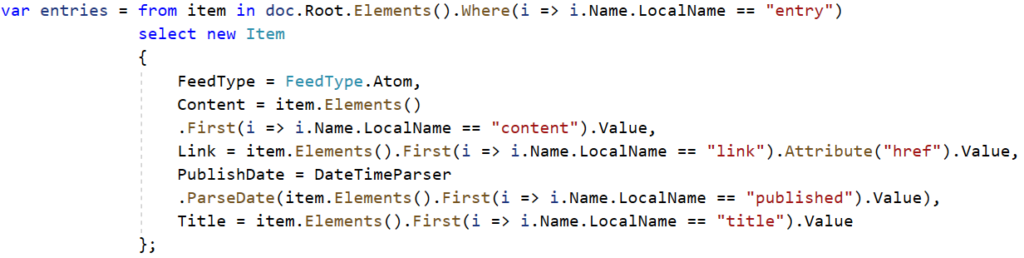
In this tutorial we saw how to parse RSS and ATOM Feeds.
How to Parse RSS and ATOM Feeds source code
You can download the full source code project from here
Next we will see how to use this Feed Parser to create a news aggregator.