So, here we are. Undo feature on Slider representing the part two of the Undo functionality implementation. If you have not read the first part, I would highly suggest that you do. Here is the link:
How to implement Undo feature on Slider Control in C# and .NET – Source Code
This time around we are not going to spend a lot of time dwelling on the C# code, because it is very similar with the one we wrote in part one of the tutorial. I will of course showcase it, but we are going to explain only the new things we are working on.
I want to mention that this tutorial series will consist of multiple parts. At the end, we are going to use our knowledge gained here to create something really cool with the Undo feature. I am still thinking in which direction I should take this series, so if you have any suggestions send it to: info@devindeep.com.
What are we going to build?
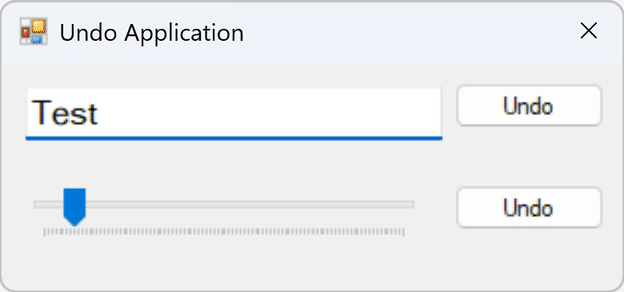
If you remember from the previous post, we build an undoable text box. This time we will create a slider with an Undo function on it. But this time, the slider will affect the text box. It will set the font size size of the text. So as a result, as you are dragging the slider button on the right, the text will increase in size.
You can also spot two Undo buttons. Well, for now they are going to be separate. At the end of the tutorial we will merge the undo functionality into a single operation. Be patient and you will find out how to do just that. But, right now we will have a separate Undo button for the text box and one for the slider.
One thing I want to mention here is the definition of the word when i say slider. Although this is technically a slider control, if you are working in WinForms it is called a TrackBar. The point here is not the control, but rather the logic behind the Undo feature. Same logic can be used to create an Undoable Slider control as well.
More DevInDeep Tutorials:
- How to Implement Face Recognition in Java
- Discover Groups with K-Means in C#
- Image Segmentation using K-Means in C#
Undo feature on Slider
First, you will need to add a new Class. Call it UndoableTrackBar. Make sure this class inherits from TrackBar control like so:
public class UndoableTrackBar : TrackBar { }
Now, let’s look at this class implementation:
public class UndoableTrackBar : TrackBar
{
public CommandController Command { get; private set; } = new CommandController();
public UndoableTrackBar()
{
ValueChanged += UndoableTrackBar_ValueChanged;
MouseCaptureChanged += UndoableTrackBar_MouseCaptureChanged;
}
private void UndoableTrackBar_ValueChanged(object sender, EventArgs e)
{
Command.Add(new TrackBarValueChangedCommand(this, Value));
ValueChanged -= UndoableTrackBar_ValueChanged;
}
private void UndoableTrackBar_MouseCaptureChanged(object sender, EventArgs e) => Command.Add(new TrackBarValueChangedCommand(this, Value));
}
As you can see from the C# code snippet above, the code is pretty similar to the one we wrote for the Undo feature for TextBox control. The first thing I want to explain here is the “UndoableTrackBar_ValueChanged” method. We subscribe to ValueChanged event to get the initial value for the TrackBar control. If we don’t do this then we won’t know the starting position-value of it. Once, we have the initial value we add it to our Command stack. Then we unsubscribe from the event, because the action I want to record in my stack is only when the user changes the value.
Of course, you can devise your own logic for ValueChanged event, but that is all up to you. Right now I want to pick up only when user takes an action on the TrackBar. So, to do that we subscribe to MouseCaptureChanged event. I would suggest to you to play with both events and see the difference. Then devise your own solution.
TrackBarChangedCommand
TrackBarChangedCommand is a separate class that implements the ICommand interface. You probably remember the other two commands we implemented for the TextBox. Well, this is quite similar. Here we execute the code and prepare the Undo operation. Here is the C# implementation:
internal class TrackBarValueChangedCommand : ICommand
{
private readonly int value = 0;
private readonly UndoableTrackBar trackBar;
public TrackBarValueChangedCommand(UndoableTrackBar trackBar, int value)
{
this.trackBar = trackBar;
this.value = value;
}
public void Execute() => trackBar.Value = value;
public Optional<ICommand> Undo() => Optional<ICommand>.Create(new TrackBarValueChangedCommand(trackBar, value));
}
There is not much to discuss here, if you have read the previous tutorial. We simply set the Value property and return the Undo command when invoked.
Wrapping it all up
The only thing left to do here is to build the solution and place the newly created control onto our Form Designer. The application should look something similar to this:
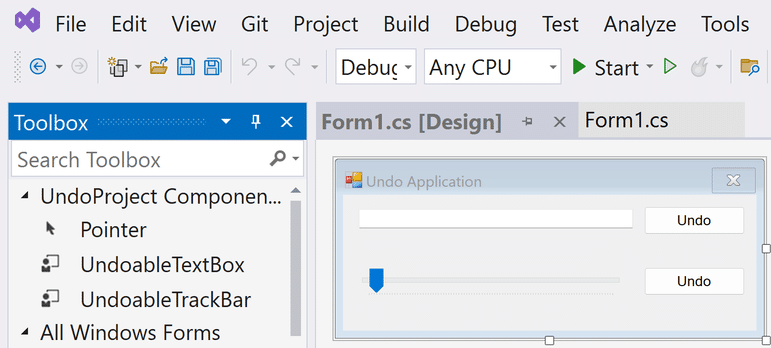
As you can see on the left side of Visual Studio there is a control named: UndoableTrackBar that you can drop onto the Windows Form. Once dropped here are some of the properties I have set:
Maximum: 100
Minimum: 5
Value: 8
These properties are set by me. I want the minimum font size to be 5. The maximum 10 and the starting value 8. Please note that you can change this at any time, but make sure the change is reflected in the Undo logic as well. One more note is that the Minimum value should not be set to 0 or less. You will get an exception because the minimum size of the font can be 1.
With all of that cleared, we are ready to dive into the C# code of the Windows Form:
public partial class Form1 : Form
{
public Form1() => InitializeComponent();
private void btnUndo_Click(object sender, EventArgs e) => txtText.Command.Undo();
private void btnUndoTrackBar_Click(object sender, EventArgs e) => trackBar.Command.Undo();
private void trackBar_ValueChanged(object sender, EventArgs e) => txtText.Font = new Font(txtText.Font.FontFamily.Name, trackBar.Value);
}
As you can see when clicking the Undo button for the slider we execute the Undo method under the TrackBar.Command class. Very similar to what we did for the TextBox. I left this intentionally because in a later tutorial we will see how these things are important. But, not right now.
Another event you can see me I have subscribed is the ValueChanged event on the TrackBar control. As a result, when the user clicks on the track and moves it left and right the font size will change. Now, when we execute the undo operation (see above) we set the Value property. This in turn fires up the ValueChanged event and sets the font size of the TextBox accordingly.
Important thing to note when implementing Undo feature is to make sure you have a strategy to solve this problem. You can solve it in another way, but it all depends on you.
Conclusion: Undo feature on Slider
This wraps up the second part of this tutorial series. Next, we will see a more complex scenario of Undo implementation. Finally, we will connect everything together in a simple up demonstrating its Undo capabilities.