This article will demonstrate the last step of a machine learning pipeline. Today we will see how to train ML.NET Model using the provided API.
ML.NET provides several training algorithms to train for different Machine Learning needs. For each task, there is a component called trainer.
Training process is very important. Combined with the evaluation assessment, we will conclude this tutorial series on introduction to ML.NET and continue investigating these concepts on a deeper level.
Trainers allow us to make sense of the data. Gain a more meaningful and valuable insights on the data set we are providing.
In other words, they learn from the data. Machine Learning algorithms are able to find relationships, develop understanding and make decisions based on the input they are given.
This is why in the last post on how to transform data we said: “Garbage In, Garbage Out”. In fact, the quality and quantity of your training data is as important as the algorithm you are using. It can make or break a Machine Learning project.
Before we start with examples on how to train ML.NET model using the API, let’s first talk about Machine Learning algorithms.
Machine Learning
Why the need for Machine Learning? Especially if we can write a set of instructions to resolve any problem we have.
In mathematics and computer science, an algorithm is defined as a finite sequence of well-defined, computer implementable instructions, typically to solve a class of problems or to perform a computation – Wikipedia.
Why not simply use algorithms?
The problem with algorithms is that they are defined as a set of instructions. And that is fine for solving a problem that follows the exact rules an algorithm defines.
The problem arises when there are simply too many variances in the problem we are trying to solve.
Let’s look at an example.
If we write a fixed set of rules to recognize the following image of a cat
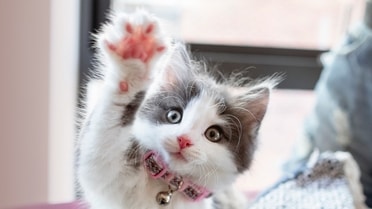
Can the algorithm recognize this image as well?
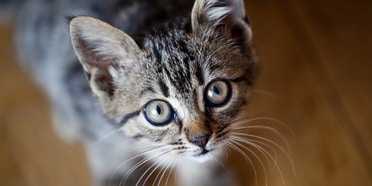
Most definitely not. Although there is a cat on both images, you would need a new set of rules to recognize cat on the second image. That is because the fur has a different color, and the cat position is different. And don’t forget the background as well as other factors such as image perspective and quality.
Imagine writing a new set of rules to detect all the different combinations that cat can appear on an image. It is simply impossible and impractical.
Patterns
But there are some distinctive patterns. We humans know it is a cat. Because of a common set of features cat species have in common.
Maybe the whiskers, eyes or the shape of the ears, eyes, and mouth reminds us of a cat.
Well, there’s our answer. Machine Learning algorithms work with data so that they can learn what makes a cat – a cat. It tries to learn cat like features just as we humans do.
Because they are able to learn complex patterns from the data, there is no need to write rules. It can deduct its own “rules” from the given input.
As a result, Machine Learning algorithms build a model based on sample data, in order to make predictions or decisions without being explicitly programmed to do so.
Machine Learning Algorithms
There are three broad categories of machine learning algorithms.
Supervised Learning
Supervised Learning algorithm requires an example inputs (training data), and their desired outputs. Think of it as a Y=f(x). The objective of these types of algorithms is to learn a target function (f) that best maps input variable (x) to an output variable (y).
They are called supervised because for each training data point you are providing a desired output. The algorithm will try to make sense of the relationship and map that input as closely as possible to the provided output.
A good example would be Cat Vs Dog Classifier. We provide a dataset which contains labeled images of dogs and cats. Labelled dataset would mean that for each input image, there is a label associated with it.
Then a supervised learning algorithm will try to learn distinct cat and dog features, so that it will be able to differentiate between them.
Unsupervised Learning
Unsupervised Learning algorithm doesn’t need labels. The dataset only contains inputs. These types of algorithms are trying to find structure in the data, like grouping or clustering. They uncover commonalities or patterns if you will, from the data itself.
Some use cases for unsupervised learning include understanding of different customer groups also known as customer segmentation, clustering DNA patterns in genetics. Recommender System which involves grouping together similar users or similar behavior. Credit card companies uses this type of algorithms to detect credit card frauds by using Anomaly Detection as a strategy, and the list goes on.
Reinforcement Learning
Reinforcement Learning algorithms acquire information about stimuli, actions, and context that predict positive outcomes. It modifies behavior when a reward occurs, or if the outcome is better than what we were expecting.
Reinforcement Learning is like teaching your dog to do tricks. You provide the treats as a reward when the dog does what it is meant to.
It is a group of Machine Learning algorithms that enables an agent to learn in environment using feedback from its own actions and experiences. In other words, the goal is to make a sequence of decisions that would maximize the reward.
ML.NET Trainers
ML.NET offers wide variety of trainers. For each Machine Learning task ML.NET provides a component that executes the training algorithm.
Each trainer can be located in their respective types. For example, the Binary Classification trainers are located under the MLContext instance BinaryClassification property.
mlContext.BinaryClassification.Trainers
On this blog we will constantly use different components to train ML.NET model. At the moment the important thing to remember is that a trainer is added as the last step in the machine learning pipeline.
So far, we have gone through tutorials on how to load data, transform it and finally we are at the end. Pass it to an algorithm that takes in a data view to train ML.NET model. Once trained we can use this model to predict future values.
One more important thing to note is that each learning algorithm is different. It takes different parameters as inputs called hyperparameters. Don’t worry too much about it for now because I will demonstrate some very simple examples.
The important part is to know where to find the ML.NET trainers and how to append them to your machine learning pipeline. In future post we will discuss different algorithms, how they work and their respective hyperparameters.
Train ML.NET Model on Regression Problem
Our first example will be to solve a regression problem.
Regression is a statistical method which attempts to determine the strength of the relationship between one dependent variable (Y) and a series of other independent variables (X1, X2, …)
A good small regression problem that we will try to solve is a bike rental scenario.
Bike Rental Scenario
Imagine you are a bike rental shop owner, and you need to know how many bikes you need to have ready at the shop. You need this kind of application because at any given time some of the bikes need to be repaired. As a result, if it is a slow day, you may send more bikes for maintenance or repair. But, if the application predicts a busy day at the shop, then you might need more bikes ready than usual.
In this scenario the number of bikes in the shop is represented by the Y variable. This variable is dependent on other variables such as temperature, season, windspeed and so on.
It is expected that a busy day in the shop is when the weather is good, it is not a workday in the summer season. When it rains or when it is cold very few bikes are being rent out.
Before setting up the model, we need data.
The Dataset
The dataset we are going to use is called: Bike Sharing Data Set and can be download from the following link.
UCI Machine Learning Repository: Bike Sharing Dataset Data Set
The Dataset is accompanied with two files. Bike rentals per day and per hour. We will use the data generated daily.
It is a CSV file so it should be easy to load. The important thing to note is that we will not attempt to solve this problem completely. The idea is to demonstrate how a complete machine learning pipeline is built.
Because of that we will predict the number of bike rents per day on the following input variables:
- Season (1: spring, 2: summer, 3: fall, 4: winter)
- Holiday (1: it is a holiday, 0: it is not a holiday)
- Weekday
- Working Day
- Weather Situation
- Clear, Few clouds, Partly cloudy
- Mist + Cloudy, Mist + Broken Clouds, Mist + Few Clouds, Mist
- Light Snow, Light Rain + Thunderstorm + Scattered Clouds, Light Rain + Scattered Clouds
- Heavy rain + Ice pallets + Thunderstorm + Mist, Snow + Fog
- Temperature
- Humidity
- Windspeed
And we will try to predict the total number of bike rentals (Casual and Registered)
So, let’s get started.
Bike Rental Shop Code Walkthrough
Everything in ML.NET starts with creating an instance from MLContext class.
var mlContext = new MLContext();
Next, we need to load the data. If you are not sure how to load data in ML.NET you can check the following tutorial.
var data = mlContext.Data.LoadFromTextFile<InputModel>("day.csv", separatorChar: ',', hasHeader: true);
The input model is defined as:
public class InputModel
{
[ColumnName("Season"), LoadColumn(2)]
public float Season { get; set; }
[ColumnName("Year"), LoadColumn(3)]
public float Year { get; set; }
[ColumnName("Month"), LoadColumn(4)]
public float Month { get; set; }
[ColumnName("Holiday"), LoadColumn(5)]
public float Holiday { get; set; }
[ColumnName("WeekDay"), LoadColumn(6)]
public float WeekDay { get; set; }
[ColumnName("WorkDay"), LoadColumn(7)]
public float WorkingDay { get; set; }
[ColumnName("Weather"), LoadColumn(8)]
public float WeatherSituation { get; set; }
[ColumnName("Temperature"), LoadColumn(9)]
public float Temperature { get; set; }
[ColumnName("Humidity"), LoadColumn(11)]
public float Humidity { get; set; }
[ColumnName("WindSpeed"), LoadColumn(12)]
public float WindSpeed { get; set; }
[ColumnName("Count"), LoadColumn(15)]
public float TotalBikeRentalCount { get; set; }
}
Now let’s build the pipeline
var pipeline = mlContext.Transforms.Concatenate("Features", new string[] { "Season","Year", "Month", "Holiday", "WeekDay", "WorkDay", "Weather", "Temperature", "Humidity", "WindSpeed" })
.Append(mlContext.Regression.Trainers.FastTree("Count", "Features"));
There is one transformation which concatenates the input values into a single vector with length of 10. If you are not sure what data transformation is, then refresh your knowledge on the following tutorial.
Because this is a regression problem, we will find the appropriate algorithm under Regression.Trainers.
ML.NET Linear Regression Trainer
The best suited trainer for this type of setup is the FastTree algorithm. All you have to know right now is that is part of the Linear Regression family of algorithms.
Also, you will notice that not all columns are included. This is because some of the features are positively correlated. For example, temp and atemp are highly positively correlated to each other. Statement like that means that they both are carrying the same information. As a result, I decided not to use atemp column.
Columns like total_count, casual, and registered are also highly positively correlated to each other. So, we are going to ignore casual and registered as well.
The last couple of paragraphs are just explaining why some of the columns are missing in my code. Right now, don’t worry about how I decided not to include them. We’ll get there. For now, I just wanted to offer a simple explanation and that’s it.
You may also ask why I chose FastTree algorithm. Well in my opinion this works best for this scenario. Please feel free to experiment with other trainers as well, and see what kind of results you get.
Remember this post is about ML.NET trainers and how to train ML.NET model. The important thing to remember here is that trainers are added as the last component to a machine learning pipeline. Also, the trainer you are going to choose depends on the machine learning task you are trying to solve.
That’s it.
We will have a dedicated tutorial on which data transformation we will apply and why. Data exploration also plays a huge role in the process as well. But we will visit these subjects in another post.
Next, we simply call the Fit method.
var model = pipeline.Fit(data);
And to get the predictions we execute the Transform method.
var predictions = model.Transform(data);
ML.NET Model Evaluation
Once you learn how to train ML.NET model, you also need to learn how to evaluate the results. Evaluation is a more elaborate process that what I will present here but let’s see a simple way to do just that.
var metrics = mlContext.Regression.Evaluate(predictions, "Count", "Score");
Console.WriteLine($"Root Mean Squared Error: {metrics.RootMeanSquaredError:#.##}");
Console.WriteLine($"RSquared Score: {metrics.RSquared:0.##}");
These metrics show the error our ML.NET Model makes. The comparison is between what we expect to get and what the model outputs. As you can see, we are comparing the Count column from the dataset with the predicted values in the Score column.
This is not a proper evaluation. We will talk about it in a dedicated post, and we will also explain the metrics that were used.
This post is already too long, so let’s end it here.
Conclusion
This article demonstrates how to train ML.NET model using the API. The framework provides various trainers for different types of Machine Learning scenarios.
What is important to note is that the trainer is appended at the end of the pipeline. Also remember that you must choose the appropriate trainer for the specific machine learning task you are trying to solve.
We saw how to use FastTree algorithm to solve a Linear Regression problem. You could also try SDCA to solve the bike rental problem.
There are also unsupervised trainers that ML.NET offers, like KMeansTrainer.
In the family of Binary Classification Trainers, you can find FastTreeBinaryTrainer and LinearSvmTrainer.
There are many other training components that I have not mentioned. We will get to them eventually with different types of hands-on projects.
Since, now we know how to train ML.NET Model we can start working on more complex scenarios and dive deeper into ML.NET.
Previous Post: How to Transform Data in ML.NET
Next Post: How to Preprocess Data in ML.NET
The source code for this tutorial can be downloaded from the following link: