In this post we are going to learn how to create AutoComplete TextBox in C#.
The focus of this tutorial will be to demonstrate the autocomplete features in Windows Forms TextBox. Born in 2003 this technology was declared as dead by Microsoft in 2014. However, WinForms is still alive and well.
Windows Forms is a UI framework for building Windows desktop applications. It provides one of the most productive ways to create desktop apps based on the visual designer provided in Visual Studio. There are many legacy projects using Windows Forms, and I am sure that almost all of them are using the TextBox control.
And that is why, we are going to complete a simple tutorial that many of you might find it useful.
What is AutoComplete TextBox?
The AutoComplete TextBox provides suggestions while you type into the field. This control enables users to quickly find and select from a pre-populated list of values as they type, leveraging searching and filtering.
Windows Forms doesn’t provide this type of control right out of the box. But, it does provide a way to achieve the desired result. To do that, we need to look into the classic TextBox control. With some small tweaks, we can transform this traditional text editing component into something more useful to us.
AutoComplete TextBox Example
In this post, I will guide you through a couple of different scenarios on how to create AutoComplete TextBox in C#. But, before we start playing with the many different options, we first need to define a goal. It is much more fun and easy to learn when you define an objective. So let’s do that now.
The Objective
I bet you have already seen how an AutoComplete control works. You have seen it on the web, or see it implemented into various desktop applications. User starts typing, and the control presents the user with several suggestions. That is exactly what we are going to build today.
We will keep it simple. We will add a field that the user needs to populate. They will enter the name of their country. As the user starts typing the TextBox control will try to help the user by presenting various suggestions.
Although this problem can be solved by using a ComboBox, for the purposes of this tutorial we will implement the solution with TextBox. And here’s how we are going to do that.
First create a new Windows Forms Solution. Make sure you use C# as the language of choice. Once the project is loaded drag and drop one Label and TextBox control onto the Windows Form.
Change the Text property of the Label to something more descriptive like “Country”. Next, we need to download the list of countries we are about to use. You can fetch a list of strings from the following link. We need this list, because we are going to use it to generate the suggestions.
AutoComplete Feature in Windows Forms
Auto completion is a new feature added to Windows Forms 2.0. So make sure you use the latest Windows Forms controls. Otherwise you will not be able to locate the properties we are about to discuss. But, even if you are on a legacy project, I will make sure to create additional tutorial on how to implement this yourself.
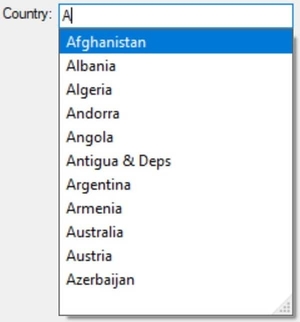
Please note that by default the TextBox control doesn’t behave this way. We need to set up a couple of things first. If you want more information, please refer to the following documentation link.
Microsoft describes the TextBox as a control that user can utilize to display, or accept input, as a single line of text. The component does a lot more, but we will focus on its AutoComplete features. As a side note, I want to mention that we can easily implement this characteristic ourselves as well. And maybe we will, if we need some more advanced options. But, for now we will simply use the out of the box solution provided by .NET.
The AutoComplete TextBox Properties
Like I mentioned previously, a TextBox control doesn’t use any of its AutoComplete features by default. To make use of them, we must use the three properties provided by Windows Forms .NET. We can set them via C# code or through the Properties window in Visual Studio.
So, select your text box, and try to locate the following properties:

Now, let’s investigate them one by one.
AutoCompleteCustomSource
Gets or sets a custom StringCollection to use when the AutoCompleteSource property is set to CustomSource.
Microsoft Documentation
When you need to generate a custom list of suggestions, this is the field you want to initialize. In our little demo, we do set this property to the Countries list. You can do that from the Visual Studio Properties window or through C# code.
If you click on the AutoCompleteCustomSource property, it will open the String Collection Editor, where we can add our strings. For example:
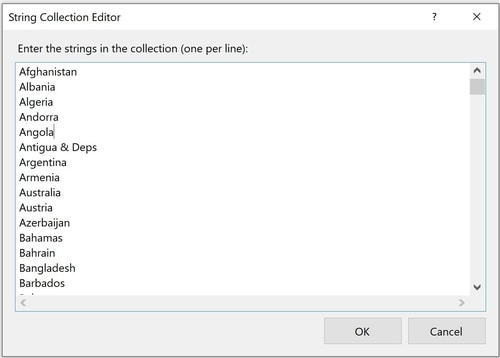
As you can see, I copied and paste the country list here. You can create your own list if you like. But, the important thing to remember is that, this is the property you set to your custom suggestion list.
Now, in order for this to work, we also need to set the AutoCompleteSource property to CustomSource. We will discuss this in the next section.
But before we do that, let’s look at the C# code that does the same exact thing as the windows forms visual editor.
var countries = File.ReadAllLines("Countries.txt");
var countriesAutocompleteCollection = new AutoCompleteStringCollection();
countriesAutocompleteCollection .AddRange(countries);
txtCountry.AutoCompleteSource = AutoCompleteSource.CustomSource;
txtCountry.AutoCompleteCustomSource = countriesAutocompleteCollection;
As you can see from the code block above, we are reading all the lines from our Countries.txt file. This file contains the list of countries separated by a new line. As a result, when we read all lines, It returns an array of strings that we are going to be using to set the AutoCompleteStringCollection.
We use the AddRange function because it accepts an array of strings. If you want to enter the suggestions one by one, then by all means do use the Add method.
Now, let’s see how the AutoCompleteSource plays into all of this.
More DevInDeep Tutorials:
- Learn How to Send Keys to Another Application
- Get Active Window Tutorial
- How to Create Transparent Image
- How to Create Controls with Rounded Corners in C#
AutoCompleteSource
Gets or sets a value specifying the source of complete strings used for automatic completion.
Microsoft Documentation
This field is an enum that specifies the source for ComboBox and TextBox automatic completion functionality. Let’s see what options can we choose from.
AutoCompleteSource Enum Table
AllSystemSources | 7 | Specifies the equivalent of FileSystem and AllUrl as the source. This is the default value when AutoCompleteMode has been set to a value other than the default. |
AllUrl | 6 | Specifies the equivalent of HistoryList and RecentlyUsedList as the source. |
CustomSource | 64 | Specifies strings from a built-in AutoCompleteStringCollection as the source. |
FileSystem | 1 | Specifies the file system as the source. |
FileSystemDirectories | 32 | Specifies that only directory names and not file names will be automatically completed. |
HistoryList | 2 | Includes the Uniform Resource Locators (URLs) in the history list. |
ListItems | 256 | Specifies that the items of the ComboBox represent the source. |
None | 128 | Specifies that no AutoCompleteSource is currently in use. This is the default value of AutoCompleteSource. |
RecentlyUsedList | 4 | Includes the Uniform Resource Locators (URLs) in the list of those URLs most recently used. |
AutoComplete TextBox File System Example
We’ve seen the CustomSource property in action. Let’s see what happens if we choose the FileSystem instead.
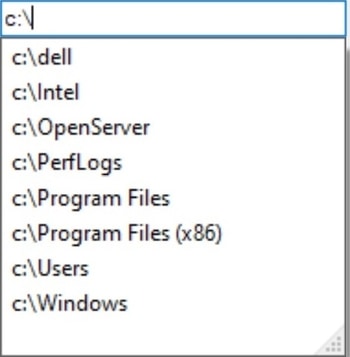
As you can see, the moment I start typing a valid file system address, the TextBox control starts suggesting locations. This is quite convenient since all I had to do is change the AutoCompleteSource property.
Let’s see how to use this in a C# code
txtFilePath.AutoCompleteSource = AutoCompleteSource.FileSystem;
If you only want to list directories, then use the AutoCompleteSource.FileSystemDirectories instead. As you can see this control AutoComplete mode works as a “Starts With” operation. What if you would like to operate as a “Contains” operation? In that case you need to implement this feature yourself.
You can easily inherit from the TextBox control and implement events such as KeyUp or KeyDown to process the user entry. You can also add a custom property to your TextBox, such as SuggestionsList and hook it up with the key handler.
The possibilities are endless and we may need to write some custom C# code to process more specific requirements. But, that is a whole other post entirely.
AutoCompleteMode
Gets or sets an option that controls how automatic completion works for the TextBox.
Microsoft Documentation
We set this property to tell the TextBox how we want the autocomplete feature to behave while the user is typing in.
None
By default this property is set to None. If it is set like this, then the AutoComplete feature is turned off.
Suggest
Displays the auxiliary drop-down list associated with the edit control. This drop-down is populated with one or more suggested completion strings. And the final result looks something like this:
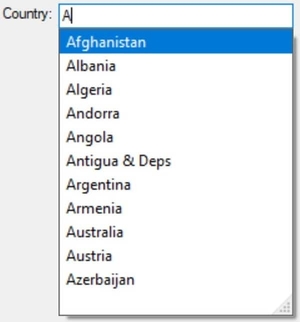
Append
Appends the remainder of the most likely candidate string to the existing characters, highlighting the appended characters.

SuggestAppend
This property is a combination of the Suggest and Append property. So it shows the drop down list of suggestions but it also appends the first string from the suggestion list. And, if you choose to use this option then you can expect your control to look something like this:
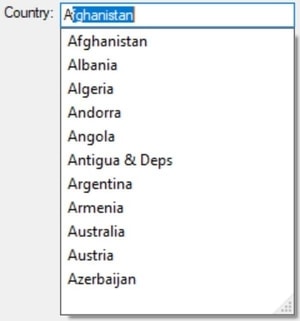
AutoComplete TextBox in C# – Conclusion
And that is all there is to it. I know some of you will face some more complex scenarios, and hopefully you will be able to solve it. Remember that you can also inherit from TextBox and implement a custom C# feature yourself. I do want to create a tutorial that will change how suggestions are matched against the list, but we will leave that for another time.