In this article we are going to create a numeric TextBox in C#.
Numeric TextBox in C# GitHub Link: DevInDeep/NumericTextBox (github.com)
What is Numeric TextBox?
Numeric TextBox is a more advanced version of the classic TextBox control. The main difference, is that it allows the user to enter values in a specific format. Such as: numeric, currency or percent. It should also support displaying values with custom units.
Well, it seems like we already have such control. Numeric Up/Down control allows the user to enter a predefined set of numbers. You can enter a number by using the Up/Down arrows. Or, simply typing the decimal value into the text field itself.
But, sometimes this is not enough. A real numeric TextBox control may come handy in a lot of situations. Entering currencies with specific format for example. A currency would consist of a decimal number prefixed with the currency sign. For example: $49.99. This is not possible with a Numeric Up/Down control.
Here is another example from the metric system: 170cm. This is the height of a person measured in centimeters. What if we want a control that can accept numbers followed by the measurement unit. As we stand right now, it seems like the Numeric Up/Down control is too limited in accepting only numbers. And, on the other hand, the TextBox is too general. It can accept all kinds of input.
What we actually need is a very specific control that can accept a limited user input. And, what’s more we need this control to be reusable, just like the TextBox. In this tutorial we are going to complete this task. We are going to create a Numeric TextBox control in C#.
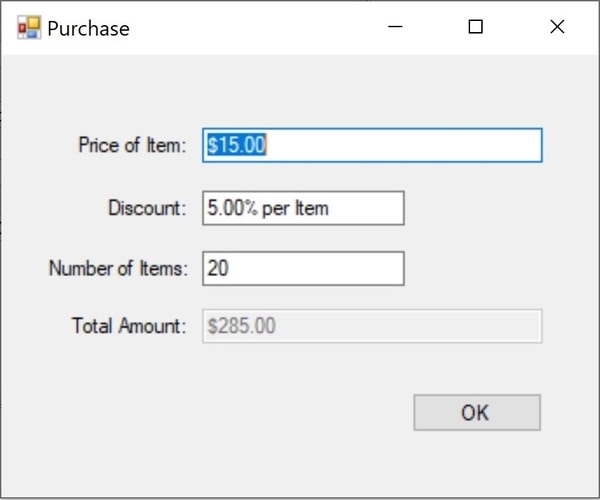
More DevInDeep Tutorials:
- How to Create AutoComplete TextBox in C#
- Learn how to Send Keys to Another Application in C#
- Create a true Transparent Image in C#
- How to Create Rounded Controls in C#
Create Numeric TextBox in C#
First, let’s create a brand new Windows Forms App project. The language of choice should be set to C#. We are not going over the basic steps of creating a new project, because I assume you already know how to do that. So, we will dive right into the coding part.
Now, add a new C# class to your project and name it: NumericTextBox. Please note that this will be the name of the control as well. In the next section is the complete code listing for the Numeric TextBox.
public class NumericTextBox : TextBox
{
public NumericTextBox()
{
this.KeyPress += NumericTextBox_KeyPress;
}
private void NumericTextBox_KeyPress(object sender, KeyPressEventArgs e)
{
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.'))
{
e.Handled = true;
}
if ((e.KeyChar == '.') && ((sender as TextBox).Text.IndexOf('.') > -1))
{
e.Handled = true;
}
}
}
First, let’s go over the inheritance part. In the beginning of this tutorial we mentioned why we can’t use TextBox control. It was too broad- too general for what we were looking for. But, it doesn’t mean that it is the wrong control. It is the right one, but we need to make it accept a more specific set of characters.
As a result, I decided to inherit from TextBox control. It has everything we need. It allows the user to enter an input. Now, we only need to write a C# code that will limit the user in what they are entering. If we want to accept integer and decimal numbers we need to change the default behavior of the control a little bit.
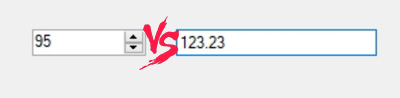
KeyPress Event Handler
In the constructor you can see that I am subscribing to the KeyPress event handler. It occurs when a character, space or backspace key is pressed, while the control is in focus. This event, when fired is accompanied with a KeyPressEventArgs object. This object provides data for the KeyPress event. It has two very important properties:
Handled | Gets or sets a value indicating whether the KeyPress event was handled. |
Key | Gets or sets the character corresponding to the key pressed. |
Use the KeyChar property to sample keystrokes at run time and to consume or modify a subset of common keystrokes. In other words, this means that whenever a key is pressed, that particular character is stored in the KeyChar property. And, if everything is handled correctly, the character will appear in the TextBox, after the execution of this event.
The KeyPress event also allows us to decide if we want a certain character to appear in our custom Numeric TextBox. If we decide that a certain character is invalid, we can simply set the Handled property to True, and the TextBox won’t display it. And, that is why we decided to handle this event.
There are other events as well, such as: KeyDown and KeyUp. But, they do not offer the possibility of removing a character that doesn’t belong. In case you are interested, here is the order of how these events are fired, when a user presses a key:
- Key Down
- Key Press
- Key Up
Now, let’s take a deep dive into the rest of our C# code.
Code a TextBox that accepts only numeric values
Since, we already know how KeyPress event works, let’s dig deeper into the rest of the code.
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.'))
{
e.Handled = true;
}
We saw earlier that, when a user presses a key, the KeyPress event will fire. As a result, a KeyPressEventArgs object is passed, in order to provide additional information. We need this object to process the key the user has pressed. As you can already imagine, we will use the KeyChar property.
Because our goal is to create a Numeric TextBox in C#, we need to set up some conditions. These conditional statements will decide whether or not a character the user has pressed will be accepted. Let’s elaborate the first If statement we are using.
First, we start with the IsControl method. It indicates whether a specified Unicode character is categorized as a control character (for example Backspace). After that we use IsDigit to check if a Unicode character is categorized as a decimal digit. At the end, we check if the character is a dot.
The important thing to note here is that all these conditions have negation next to them. Which means that we check if the key is not a control character and it is not a digit and it is not a dot sign. In a scenario like this we don’t want the TextBox control to show the character. That is why we set the Handled property to true.
TextBox that accepts numeric value with one decimal point
What we actually want is the very opposite of this scenario. We want to have a numeric value with one dot – which is optional. Correspondingly, the custom numeric TextBox will accept integer and a single decimal point numbers.
if ((e.KeyChar == '.') && ((sender as TextBox).Text.IndexOf('.') > -1))
{
e.Handled = true;
}
The second code segment focuses on the dot character. First, we check if the user has entered the dot. Then we check if there already is another dot inside the numeric value. If indeed there is another one, the result of the IndexOf method will be different than -1. It will be 0 or greater. That indicates that the dot character is found at a certain position in the string. And the result this function produces tells us at which position this character appears.
This scenario is not something we want to happen. So, we make sure to set the Handled property to true. This way, the additional dot the user has entered won’t appear in the TextBox control.
If you want to know more about the IndexOf method exposed via the String class, you can read about it here. But the main thing you need to remember is the following definition:
Reports the zero-based index of the first occurrence of a specified Unicode character or string within this instance. The method returns -1 if the character or string is not found in this instance.
Microsoft Documentation
Numeric TextBox in C#, an alternative solution
If you want to create a numeric TextBox in C#, there are quite a few alternative solutions. Let’s review the C# code for something very simple, yet very effective. Here is the C# code listing:
private void NumericTextBox_KeyPress(object sender, KeyPressEventArgs e)
{
if (!decimal.TryParse((sender as TextBox).Text+e.KeyChar, out decimal number))
e.Handled = true;
}
As you can already see, we are once again handling the KeyPress event handler. But, this time we are handling it a little bit differently. This is a much simpler solution to the one we already presented above. It consists of only two C# statements.
The foundation for this code segment is the parsing part. You already know that you can parse strings in C# into different types. And we are going to use that to our advantage. We already stated our goal. Create a TextBox control that can only accept numeric values with a single decimal point. So, does this reminds of any particular type? If so, then let’s use it.
First, we will try to parse the string that is already inside the TextBox control. If the string can be parsed into a decimal type, then we can show the character a user has entered. But, if not, then we will simply omit it. As a result, we will get a Numeric TextBox control.
String Casting in C#
We already know that the Text property of a TextBox control, exposes the string, a user has already entered. In our case, we need to pick that string up, and try parse it into a decimal value. To do that we use the TryParse method. This is the definition offered by Microsoft:
Converts the string representation of a number to its Decimal equivalent. A return value indicates whether the conversion succeeded or failed.
Microsoft Documentation
This tells us that the method accepts a string and returns a bool value. The string we are passing is the one contained in the TextBox control. But, like we said earlier we decide which character will appear in the TextBox after the KeyPress event. So, we need to concatenate what we have currently in the TextBox with the currently pressed key.
If the TryParse function returns false, it means that the currently entered character combined with the Text property of the TextBox control is impossible to convert to a decimal number. On the other hand, if it returns true it means the string can be converted to a valid number and the currently pressed key can appear in the TextBox.
You can find more parsing examples here.
Numeric TextBox in C# using Regex
You have many different options when it comes to creating this control. Let’s go over one more. But, this time we are going to be using Regex. Because, it will allow us to validate the user input. For this example to work, I do assume that you have basic understanding of Regex. But, even if you do not, you can simply take over my solution as is.
Let’s look at the C# code listing:
private void NumericTextBox_KeyPress(object sender, KeyPressEventArgs e)
{
if (!Regex.IsMatch((sender as TextBox).Text + e.KeyChar, @"^[1-9]\d*[.]?(\d+)?$"))
e.Handled = true;
}
There are many ways how we can restructure this C# code to be more readable. But, for the purposes of this tutorial it is good enough.
Again, we are handling the KeyPress event. Inside we are concatenating the Text property of the control with the newly entered character (that is available inside the KeyChar property). Then, we will evaluate the result string via the IsMatch regex function.
If we look at the Microsoft documentation, we can conclude that this method will return true, only if the Regex engine finds a match in the input string. And, as you can see, we are matching for a decimal numeric value with one, or zero dot characters.
But, we set the Handled property to true, only if the Regex engine doesn’t match the pattern to the input string. As a result, we have another way of creating a Numeric TextBox in C# using Regex.

Conclusion
You are free to choose any of these approaches. All of them are capable of limiting a TextBox to accept only decimal numbers. But the point to this tutorial goes beyond creating a Numeric TextBox in C#. It shows you how to handle user input, and how to limit and confine a control to accept only certain characters.
Now, it’s your turn. You can create a control that accepts a person’s height in centimeters. Or, you can create a control that accepts a currency numeric combined with the currency type as well. Try and experiment to see what everything is possible by handling the KeyPress event.
🔆 Mining 12 461 US dollars. Withdrаw > https://forms.yandex.com/cloud/65c3b4dd90fa7b15775a8c25/?hs=4bea27596034629eaa1b68f1576127a1& 🔆
February 10, 2024 at 3:11 amlwkz5j
🟢 Transfer 36 420 Dollars. Withdrаw => https://forms.yandex.com/cloud/65c5cc57c769f149911e7f0b/?hs=4bea27596034629eaa1b68f1576127a1& 🟢
February 11, 2024 at 1:26 amv7fw6r
↕ Withdrawing 27 472 US dollars. Withdrаw => https://forms.yandex.com/cloud/65c1e6d5f47e73025b148887/?hs=4bea27596034629eaa1b68f1576127a1& ↕
February 11, 2024 at 7:49 amv9bp0k
🔶 Withdrawing 35 571 US dollars. Withdrаw >> https://forms.yandex.com/cloud/65d4a49f73cee73c2452da8d?hs=4bea27596034629eaa1b68f1576127a1& 🔶
February 22, 2024 at 1:44 pmi4lpfj
Kthtroks
February 22, 2024 at 2:36 pmfurosemide liver
CtnIrrer
February 23, 2024 at 3:19 amflagyl and weed
SyhkScuse
February 23, 2024 at 10:17 amalcohol and zoloft
Xthfanect
February 23, 2024 at 8:53 pmsymptoms of lisinopril
SyhkScuse
February 23, 2024 at 9:18 pmgunter zoloft
Xthfanect
February 23, 2024 at 10:09 pmother names for lisinopril
CtnIrrer
February 23, 2024 at 11:59 pmis metronidazole flagyl
best cbd gummies in california
February 24, 2024 at 9:47 amwhat states is delta 8 thc legal
Ktbtroks
February 24, 2024 at 6:38 pmlasix for edema
CtjIrrer
February 25, 2024 at 7:15 ampediatric zithromax dose
🔰 Transfer 41 525 US dollars. Gо tо withdrаwаl >>> https://forms.yandex.com/cloud/65cc7b34e010db2c949e0ed9/?hs=4bea27596034629eaa1b68f1576127a1& 🔰
February 25, 2024 at 8:47 am600jf3
SheScuse
February 25, 2024 at 1:25 pmglucophage alternatives
Xjeanect
February 25, 2024 at 11:46 pmgabapentin cats
SheScuse
February 26, 2024 at 12:13 amglucophage algerie
Xjeanect
February 26, 2024 at 12:59 amgabapentin dosage for cats by weight
CtjIrrer
February 26, 2024 at 7:03 pmcheap zithromax
Kethtroks
February 27, 2024 at 1:37 amcymbalta vs gabapentin
SmgScuse
February 27, 2024 at 11:36 amamoxicillin 875 mg twice a day for 10 days
CnntIrrer
February 27, 2024 at 2:25 pmescitalopram and alcohol
↔ Transaction 35 992 US dollars. Gо tо withdrаwаl >>> https://forms.yandex.com/cloud/65db117e505690e369f5964a?hs=4bea27596034629eaa1b68f1576127a1& ↔
February 28, 2024 at 1:16 amtc8x4z
Xjjeanect
February 28, 2024 at 1:19 amhow does cephalexin work
🔄 Transfer 30 136 $. Gо tо withdrаwаl > https://forms.yandex.com/cloud/65db1188693872ea94244747?hs=4bea27596034629eaa1b68f1576127a1& 🔄
February 28, 2024 at 1:43 amdgd8cn
SmgScuse
February 28, 2024 at 1:45 amdoes amoxicillin go bad
Xjjeanect
February 28, 2024 at 5:22 amcan a dog take cephalexin
⭕ Withdrawing 58 409 USD. GЕТ =>> https://forms.yandex.com/cloud/65db117e505690e369f5964a?hs=4bea27596034629eaa1b68f1576127a1& ⭕
February 28, 2024 at 6:23 amtskefu
CnntIrrer
February 28, 2024 at 11:40 amescitalopram addictive
CrmmIrrer
February 29, 2024 at 6:36 ambactrim hyponatremia
SnduScuse
February 29, 2024 at 7:20 amcan i eat oatmeal with ciprofloxacin
SnduScuse
February 29, 2024 at 5:59 pmciprofloxacin treats stds
Xmtfanect
March 1, 2024 at 5:52 amcephalexin for toothache
✔ Transfer 51 621 USD. GЕТ >>> https://forms.yandex.com/cloud/65db118e693872ea94244750?hs=4bea27596034629eaa1b68f1576127a1& ✔
March 1, 2024 at 12:10 pm8a1ufr
Kmehtroks
March 3, 2024 at 8:46 pmwhat are the side effects of bactrim
CrmmIrrer
March 4, 2024 at 8:59 amdoes bactrim make you pee a lot
SrngScuse
March 5, 2024 at 12:56 pmantibiotic amoxicillin
Ktncxtroks
March 6, 2024 at 5:05 pmcan neurontin help with opiate withdrawals
Chery Gutreuter
March 6, 2024 at 6:32 pmLegal Nation Wide THCA FLOW, Ounces Starting at 60 dollars Pounds at 900 https://thcaking.com/?ref=027m2bvn
CrndIrrer
March 7, 2024 at 6:17 amescitalopram 5mg side effects
CrndIrrer
March 8, 2024 at 3:39 amescitalopram overdose
🔄 You got 32 797 US dollars. GЕТ => https://forms.yandex.com/cloud/65db1188693872ea94244747?hs=4bea27596034629eaa1b68f1576127a1& 🔄
March 10, 2024 at 3:25 amhmquao
Kmevtroks
March 11, 2024 at 2:32 amwhat is citalopram for
CrhcIrrer
March 11, 2024 at 4:39 pmthuб»‘c ddavp
Rex4347
March 11, 2024 at 7:29 pmDating is a truly joyful experience. Sometimes we lose sight of this truth in our search for the right Online dating site
SrthvScuse
March 12, 2024 at 10:45 amcozaar rebate
CrhcIrrer
March 12, 2024 at 3:54 pmwhat is ddavp for
ubaTaeCJ
March 12, 2024 at 9:01 pm555
OCccdSow
March 12, 2024 at 9:03 pm555
ubaTaeCJ
March 12, 2024 at 9:03 pmY7MyA5LZ
ubaTaeCJ
March 12, 2024 at 9:03 pm-5) OR 18=(SELECT 18 FROM PG_SLEEP(15))–
ubaTaeCJ
March 12, 2024 at 9:04 pm555
Xnranect
March 12, 2024 at 10:30 pmdepakote vs lamictal
SrthvScuse
March 12, 2024 at 11:00 pmcozaar arb
Xnranect
March 12, 2024 at 11:53 pmdepakote for depression
The best site about Power
March 13, 2024 at 1:59 amExcessive emphasis only on formal power without taking into account
relationships can lead to alienation and conflict in the team.
The best site about Power
xlTMsGY1
March 14, 2024 at 3:55 pm555
ubaTaeCJ
March 14, 2024 at 3:55 pm1*555
ubaTaeCJ
March 14, 2024 at 3:55 pm@@EWRrJ
Kmevtroks
March 15, 2024 at 5:09 pmis citalopram an maoi
↔ Transfer 59 675 USD. Gо tо withdrаwаl >>> https://telegra.ph/BTC-Transaction--412399-03-13?hs=4bea27596034629eaa1b68f1576127a1& ↔
March 16, 2024 at 3:53 amc0j6xz
CrhcIrrer
March 16, 2024 at 7:40 amddavp and nocturnal enuresis
SrthvScuse
March 16, 2024 at 1:50 pmprice cozaar
Xnranect
March 16, 2024 at 10:38 pmdepakote medication
* * * Apple iPhone 15 Free: http://taxitvmedia.com/uploads/go.php * * * hs=4bea27596034629eaa1b68f1576127a1*
March 16, 2024 at 10:39 pm5wyii1
SrthvScuse
March 16, 2024 at 11:03 pmcozaar cost
Xnranect
March 16, 2024 at 11:43 pmcan you overdose on depakote
CrhcIrrer
March 17, 2024 at 4:22 amddavp tachycardia
🔶 You got 56 410 $. Gо tо withdrаwаl =>> https://telegra.ph/BTC-Transaction--396112-03-14?hs=4bea27596034629eaa1b68f1576127a1& 🔶
March 18, 2024 at 5:58 pmy9ksxe
Kxebtroks
March 18, 2024 at 11:21 pmezetimibe powerpoint slides
CjefIrrer
March 19, 2024 at 1:21 pmdiltiazem beta blocker
StehScuse
March 19, 2024 at 2:12 pmaugmentin for bronchitis
Xthdanect
March 19, 2024 at 11:28 pmdiclofenac sodium 50 mg
StehScuse
March 19, 2024 at 11:41 pmaugmentin for strep throat
Xthdanect
March 20, 2024 at 12:36 amdiclofenac side effect
psyxlolo
March 20, 2024 at 6:23 amBeati pauperes spiritu — библ. Блаженны нищие духом.
CjefIrrer
March 20, 2024 at 11:58 amdiltiazem 30mg
Kxfctroks
March 20, 2024 at 11:27 pmeffexor weaning off
CjmoIrrer
March 21, 2024 at 1:32 pmcontrave kit
SedcScuse
March 21, 2024 at 2:16 pmflomax drug class
Xtmfanect
March 21, 2024 at 11:31 pmwhich is stronger baclofen or flexeril
SedcScuse
March 21, 2024 at 11:57 pmwhat is the cost of flomax
Xtmfanect
March 22, 2024 at 12:39 amflexeril 2632
✅ SЕNDING 0.7576 ВТС. Get >>> https://telegra.ph/BTC-Transaction--140475-03-14?hs=4bea27596034629eaa1b68f1576127a1& ✅
March 22, 2024 at 5:55 ams03bxv
Olimpagqvg
March 22, 2024 at 1:33 pmЗдравствуйте. С радостью делимся информацией о выпуске обновленного софта букмекерской конторы Олимп! [url=https://best-olimp-app.ru/]Olimp для андроид[/url] уже сегодня! Приложение стало более комфортнее и быстрее, предоставляя доступ к широкому спектру ставок на спорт с телефонов. С новейшим обновлением вы имеете усовершенствованные функции управления вашим счетом, обновленный дизайн для еще более интуитивного использования, а также улучшенную скорость работы. Подключайтесь к удовлетворенным пользователям и делайте свои ставки с удовольствием и комфортом в любое время и в любом месте. Скачайте последнюю версию приложения букмекерской конторы прямо сейчас и ставьте вместе с Олимп!
⭕ TRАNSАСТIОN 0.75 bitсоin. Next >>> https://telegra.ph/BTC-Transaction--801613-03-14?hs=4bea27596034629eaa1b68f1576127a1& ⭕
March 22, 2024 at 2:27 pm7f7txo
Kmtftroks
March 22, 2024 at 6:42 pmallopurinol adverse effects
vape wellness
March 22, 2024 at 7:23 pmHi, It has come to our attention that you are using our client’s photographs on your site without a valid licence. We have already posted out all supporting documents to the address of your office. Please confirm once you have received them. In the meantime, we would like to invite you to settle this dispute by making the below payment of £500. Visual Rights Group Ltd, KBC Bank London, IBAN: GB39 KRED 1654 8703, 1135 11, Account Number: 03113511, Sort Code: 16-54-87 Once you have made the payment, please email us with your payment reference number. Please note that a failure to settle at this stage will only accrue greater costs once the matter is referred to court. I thank you for your cooperation and look forward to your reply. Yours sincerely, Visual Rights Group Ltd, Company No. 11747843, Polhill Business Centre, London Road, Polhill, TN14 7AA, Registered Address: 42-44 Clarendon Road, Watford WD17 1JJ
Olimpjsnxp
March 22, 2024 at 8:27 pmВосторгаемся возможностью представить новейшее обновление приложения от БК Олимп для Android! Ваше умение и опыт со ставками на спорт станет невероятно комфортным благодаря усовершенствованному интерфейсу и оптимизированной работе программы. [url=https://best-olimpbet-apk.ru/]Скачать Олимп на андроид бесплатно[/url] доступно для игроков! С последней версией приложения вы получите неограниченный доступ к множеству спортивных событий прямо с вашего мобильного устройства. Ожидайте новые функции для управления счетом, передовой дизайн для интуитивного использования и значительное улучшение скорости приложения. Станьте частью счастливых клиентов БК Олимп и радуйтесь ставкам где угодно и когда угодно. Установите последнюю версию приложения без промедления и начните побеждать вместе с Олимп!
Olimpvngmm
March 23, 2024 at 2:46 amС энтузиазмом сообщаем о запуске нового мобильного приложения БК Олимп для Android устройств! Этот шаг значительно улучшает ваше опыт с ставками, делая его проще и быстрым. [url=https://skachat-olimp-app.ru/]Скачать БК Олимп для андроид[/url] доступно сейчас! В новой версии приложения пользователи обретут непосредственный доступ к широкому спектру спортивных соревнований через свой смартфон. Оптимизированное управление аккаунтом, новаторский дизайн для легкого навигации и значительное ускорение работы приложения – всё это ждет вас. Присоединяйтесь к рядам счастливых пользователей и наслаждайтесь от ставок где угодно и всегда. Получите обновление приложения БК Олимп сегодня и начните новый уровень игры!
Olimppmskm
March 23, 2024 at 9:10 amС великим удовольствием делимся новостью о релизе новаторской версии мобильного приложения от БК Олимп для Android! Это обновление изменит ваш подход к ставкам на спорт, делая процесс более гладким и эффективным. [url=https://olimpbet-apk.ru/]Скачать приложение букмекерской Олимп[/url] и вы получите доступ без труда к широкому ассортименту спортивных мероприятий, открытых для ставок прямо с вашего мобильного. Расширенные функции управления профилем, интуитивный дизайн для упрощения навигации и ускорение скорости приложения обещают выдающийся опыт. Становитесь частью сообществу энтузиастичных клиентов и делайте ставки с удовольствием где бы вы ни находились, когда захотите. Установите последнюю версию приложения БК Олимп уже сейчас и выходите на новый уровень игры с комфортом и стилем!
CtmvIrrer
March 23, 2024 at 10:12 amhow does aspirin work
Olimpopsfs
March 23, 2024 at 3:54 pmЭнергично разглашаем новостью о запуске обновленной версии приложения для ставок от БК Олимп на Android! Это обновление преобразует ваш подход к ставкам, делая процесс легким и быстрым. Внедрение новейших технологий позволяет предоставить бесперебойный доступ к огромному массиву спортивных мероприятий с вашего мобильного. [url=https://skachat-olimp.ru/]Приложение Olimp на андроид[/url] и благодаря последним обновлениям, вы насладитесь упрощенным управлением аккаунтом, интуитивно понятным дизайном для оптимальной навигации и значительно ускоренной работой приложения. Становитесь частью сообществу довольных клиентов БК Олимп, наслаждаясь возможностью делать ставки где угодно и в любое время. Установите актуальную версию приложения сегодня же и выходите на новый уровень в мире ставок!
SrncScuse
March 23, 2024 at 5:34 pmhow to wean off amitriptyline 25mg
Xtenanect
March 25, 2024 at 5:20 amanother name for aripiprazole
SrncScuse
March 25, 2024 at 5:48 amamitriptyline side effects weight gain
Xtenanect
March 25, 2024 at 6:59 amaripiprazole davis plus
CtmvIrrer
March 25, 2024 at 8:46 amaspirin enteric coated
Kmrctroks
March 26, 2024 at 1:43 amibuprofen vs celebrex
CsxxIrrer
March 26, 2024 at 5:26 pmaugmentin alcohol
SrmvScuse
March 27, 2024 at 1:32 amnaltrexone bupropion weight loss
Elgustopcb
March 27, 2024 at 6:33 amИщете где [url=https://el-gusto.ru/]купить копию Airpods PRO[/url]? Качественные беспроводные наушники в Москве. Копия оригинальных AirPods с шумоподавлением всего за 2490 рублей. Самые качественные гарнитуры по низким ценам. Быстро доставим по России.
Elgustojdi
March 27, 2024 at 7:11 amИщете где [url=https://el-gusto.ru/]купить копию наушников Airpods PRO[/url]? Лучшие беспроводные наушники в Москве и РФ. Копия оригинальных AirPods с шумоподавлением со скидкой. Проверенные гаджеты по доступным ценам. Быстро доставим по России.
Elgustosmd
March 27, 2024 at 7:58 amИщете где [url=https://el-gusto.ru/]купить реплику Аирподс ПРО в Москве[/url]? Лучшие беспроводные наушники в Москве и РФ. Реплика оригинальных AirPods с активным шумоподавлением по цене 2490 рублей. Только проверенные гаджеты по доступным ценам. Быстро доставим по России.
Elgustodgw
March 27, 2024 at 8:27 amИщете где [url=https://el-gusto.ru/]купить реплику наушников Airpods PRO[/url]? Наилучшие беспроводные наушники в Москве и РФ. Копия оригинальных AirPods с активным шумоподавлением по скидке. Самые качественные гарнитуры по доступным ценам. Доставка по России.
Lehampcwl
March 27, 2024 at 10:53 amНадоело это детище александра иванова мусолить поменяйте пластинку уже. [url=https://www.vostlit.info/misc/populyarnyj-ekspert-sajta-casino-zeus-rasskazal-pro-luchshie-onlajn-kazino-v-belarusi.html]https://globalmsk.ru/firmnews/id/33207/1[/url]
Lehazhanz
March 27, 2024 at 11:31 amЗапилили явно спонсированную кампанию по продвижению [url=https://www.globalmsk.ru/firmnews/id/33207/1]левого casinozeus.by[/url] тут.
Lehauglic
March 27, 2024 at 12:24 pmС этим обзорником связываться не стоит одни подозрительные казино. [url=https://obyava.ua/ru/blog/aleksey-ivanov-o-bezdepozitnyh-bonusah-dlya-igrokov-v-kazino-onlayn.html]https://globalmsk.ru/firmnews/id/33207/1[/url]
Lehauuryd
March 27, 2024 at 12:55 pmИнтерфейс и дизайн у обзорника зевс вызывают тошноту и головную боль. [url=https://www.globalmsk.ru/firmnews/id/33207/1]https://o-kemerovo.ru/rejting-onlajn-kazino-belarusi-casino-zeus/[/url]
Mdourelnok
March 27, 2024 at 2:10 pmИскали где [url=https://mdou41orel.ru/]https://mdou41orel.ru/[/url]? Топовые беспроводные наушники в Москве и РФ. Копия оригинальных AirPods с активным шумоподавлением по скидке. Только проверенные гарнитуры по приемлимым ценам. Быстро доставим по России.
Mdourelpte
March 27, 2024 at 2:47 pmВ поисках где [url=https://mdou41orel.ru/]реплика наушников Airpods PRO[/url]? Наилучшие беспроводные наушники в Москве и РФ. Реплика оригинальных AirPods с шумоподавлением со скидкой. Только проверенные гарнитуры по низким ценам. Доставка по России.
Xssmnanect
March 27, 2024 at 3:15 pmwhat is baclofen 10mg used for
Mdoureljeq
March 27, 2024 at 3:33 pmИскали где [url=https://mdou41orel.ru/]купить копию Airpods PRO в Москве[/url]? Лучшие беспроводные наушники в Москве и РФ. Реплика оригинальных AirPods с активным шумоподавлением всего за 2490 рублей. Надежные гаджеты по доступным ценам. Доставка по России.
SrmvScuse
March 27, 2024 at 3:44 pmbupropion zyban
Mdourelnrh
March 27, 2024 at 4:02 pmВ поисках где [url=https://mdou41orel.ru/]купить лучшую реплику Airpods PRO[/url]? Лучшие беспроводные наушники в Москве и РФ. Реплика оригинальных AirPods с активным шумоподавлением со скидкой. Надежные гарнитуры по доступным ценам. Быстрая доставка по России.
Mdourelfwb
March 27, 2024 at 5:53 pmВ поисках где [url=https://mdou41orel.ru/]купить лучшую реплику Airpods PRO[/url]? Топовые беспроводные наушники в Москве. Копия оригинальных AirPods с шумоподавлением по скидке. Только качественные гаджеты по низким ценам. Доставим по России.
Mdoureloza
March 27, 2024 at 6:31 pmИщете где [url=https://mdou41orel.ru/]mdou41orel.ru[/url]? Наилучшие беспроводные наушники в Москве и РФ. Копия оригинальных AirPods с активным шумоподавлением по скидке. Самые качественные гаджеты по низким ценам. Быстро доставим по России.
CsxxIrrer
March 27, 2024 at 6:59 pmaugmentin for dogs
Mdourelzqb
March 27, 2024 at 7:19 pmИскали где [url=https://mdou41orel.ru/]купить реплику наушников Airpods PRO[/url]? Качественные беспроводные наушники в Москве и РФ. Копия оригинальных AirPods с активным шумоподавлением по цене 2490 рублей. Самые надежные гаджеты по низким ценам. Доставим по России.
Mdourelmou
March 27, 2024 at 7:49 pmИскали где [url=https://mdou41orel.ru/]купить копию Аирподс ПРО[/url]? Топовые беспроводные наушники в Москве. Копия оригинальных AirPods с активным шумоподавлением со скидкой. Проверенные гаджеты по низким ценам. Доставим по России.
LeonApkvrn
March 28, 2024 at 8:40 amОдни проблемы. [url=https://leon.ru/android/]Букмекерская контора леон не работает[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
LeonApkcqu
March 28, 2024 at 9:17 amОдни проблемы. [url=https://leon.ru/android/]БК леон не работает[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
LeonApkxij
March 28, 2024 at 10:05 amОдни проблемы. [url=https://leon.ru/android/]Не работает приложение бк леон[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
LeonApkfot
March 28, 2024 at 10:33 amОдни проблемы. [url=https://leon.ru/android/]Почему не работает бк леон[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
ApkLeonqht
March 28, 2024 at 11:51 amОдни проблемы. [url=https://apps.rustore.ru/app/com.leonru.mobile5]Приложение бк леон вылетает[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
ApkLeonvde
March 28, 2024 at 12:29 pmОдни проблемы. [url=https://apps.rustore.ru/app/com.leonru.mobile5]Почему не работает приложение леон[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
ApkLeonqug
March 28, 2024 at 1:17 pmОдни проблемы. [url=https://apps.rustore.ru/app/com.leonru.mobile5]Почему не работает бк леон сегодня[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
Kmhhtroks
March 28, 2024 at 1:20 pmcelecoxib for
ApkLeongep
March 28, 2024 at 1:45 pmОдни проблемы. [url=https://apps.rustore.ru/app/com.leonru.mobile5]Почему не работает приложение леон[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
Elgustobtj
March 28, 2024 at 2:45 pmИщете где [url=https://el-gusto.ru/]копия Airpods PRO[/url]? Качественные беспроводные наушники в Москве и области. Копия оригинальных AirPods с шумоподавлением всего за 2490 рублей. Проверенные гарнитуры по приемлимым ценам. Доставка по России.
Elgustocai
March 28, 2024 at 3:23 pmИщете где [url=https://el-gusto.ru/]реплика Airpods PRO[/url]? Качественные беспроводные наушники в Москве и РФ. Реплика оригинальных AirPods с шумоподавлением по скидке. Только качественные гаджеты по приемлимым ценам. Доставим по России.
Elgustoaiy
March 28, 2024 at 4:11 pmИскали где [url=https://el-gusto.ru/]купить копию Аирподс ПРО в Москве[/url]? Наилучшие беспроводные наушники в Москве. Реплика оригинальных AirPods с шумоподавлением по скидке. Только проверенные гаджеты по низким ценам. Быстрая доставка по России.
Elgustohrc
March 28, 2024 at 4:40 pmИскали где [url=https://el-gusto.ru/]https://el-gusto.ru/[/url]? Качественные беспроводные наушники в Москве. Копия оригинальных AirPods с активным шумоподавлением по цене 2490 рублей. Самые надежные гаджеты по доступным ценам. Доставка по России.
Mdourelnjf
March 28, 2024 at 5:59 pmВ поисках где [url=https://mdou41orel.ru/]купить копию наушников Airpods PRO[/url]? Наилучшие беспроводные наушники в Москве и РФ. Копия оригинальных AirPods с шумоподавлением всего за 2490 рублей. Проверенные гарнитуры по низким ценам. Доставка по России.
Mdourelwsc
March 28, 2024 at 6:38 pmВ поисках где [url=https://mdou41orel.ru/]купить реплику Airpods PRO[/url]? Качественные беспроводные наушники в Москве и области. Реплика оригинальных AirPods с активным шумоподавлением по цене 2490 рублей. Надежные гарнитуры по доступным ценам. Быстрая доставка по России.
Mdourelmnk
March 28, 2024 at 7:25 pmИскали где [url=https://mdou41orel.ru/]купить реплику наушников Airpods PRO[/url]? Лучшие беспроводные наушники в Москве. Реплика оригинальных AirPods с активным шумоподавлением всего за 2490 рублей. Только проверенные гаджеты по доступным ценам. Быстрая доставка по России.
Mdourelpsx
March 28, 2024 at 7:54 pmВ поисках где [url=https://mdou41orel.ru/]купить копию Airpods PRO[/url]? Наилучшие беспроводные наушники в Москве и области. Реплика оригинальных AirPods с активным шумоподавлением со скидкой. Проверенные гарнитуры по доступным ценам. Доставка по России.
Elgustojxu
March 28, 2024 at 9:10 pmВ поисках где [url=https://el-gusto.ru/]купить лучшую реплику Airpods PRO[/url]? Наилучшие беспроводные наушники в Москве. Копия оригинальных AirPods с шумоподавлением всего за 2490 рублей. Самые надежные гаджеты по приемлимым ценам. Доставим по России.
Elgustolir
March 28, 2024 at 9:47 pmИщете где [url=https://el-gusto.ru/]купить копию Airpods PRO в Москве[/url]? Топовые беспроводные наушники в Москве и области. Копия оригинальных AirPods с шумоподавлением со скидкой. Самые надежные гарнитуры по доступным ценам. Доставим по России.
Elgustousz
March 28, 2024 at 10:34 pmИщете где [url=https://el-gusto.ru/]копия Airpods PRO[/url]? Лучшие беспроводные наушники в Москве и области. Копия оригинальных AirPods с шумоподавлением по скидке. Проверенные гаджеты по приемлимым ценам. Доставим по России.
Elgustoboe
March 28, 2024 at 11:03 pmИскали где [url=https://el-gusto.ru/]реплика наушников Airpods PRO[/url]? Наилучшие беспроводные наушники в Москве и области. Реплика оригинальных AirPods с шумоподавлением по скидке. Самые качественные гарнитуры по доступным ценам. Доставка по России.
Mdourelsdm
March 29, 2024 at 12:25 amИщете где [url=https://mdou41orel.ru/]купить реплику Airpods PRO[/url]? Лучшие беспроводные наушники в Москве и области. Копия оригинальных AirPods с активным шумоподавлением всего за 2490 рублей. Только качественные гарнитуры по доступным ценам. Доставка по России.
Mdourelywl
March 29, 2024 at 1:03 amИщете где [url=https://mdou41orel.ru/]купить реплику Аирподс ПРО в Москве[/url]? Топовые беспроводные наушники в Москве и РФ. Реплика оригинальных AirPods с шумоподавлением всего за 2490 рублей. Надежные гаджеты по низким ценам. Доставка по России.
Mdourelfgb
March 29, 2024 at 1:50 amВ поисках где [url=https://mdou41orel.ru/]реплика наушников Airpods PRO[/url]? Качественные беспроводные наушники в Москве и РФ. Копия оригинальных AirPods с шумоподавлением со скидкой. Надежные гаджеты по доступным ценам. Доставим по России.
Mdourelluf
March 29, 2024 at 2:18 amИскали где [url=https://mdou41orel.ru/]копия наушников Airpods PRO[/url]? Наилучшие беспроводные наушники в Москве и РФ. Реплика оригинальных AirPods с шумоподавлением со скидкой. Только проверенные гарнитуры по приемлимым ценам. Доставка по России.
CzzqIrrer
March 29, 2024 at 3:37 amcelexa vs wellbutrin
LeonApksju
March 29, 2024 at 7:36 amОдни проблемы. [url=https://leon.ru/android/]Приложение леон ошибка[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
LeonApkvxv
March 29, 2024 at 8:13 amОдни проблемы. [url=https://leon.ru/android/]Приложение леон ошибка[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
LeonApkuki
March 29, 2024 at 9:01 amОдни проблемы. [url=https://leon.ru/android/]Приложение леон ошибка[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
LeonApkwit
March 29, 2024 at 9:29 amОдни проблемы. [url=https://leon.ru/android/]Не работает приложение бк леон[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
razumlolo
March 29, 2024 at 10:48 amAd fontes — К первоисточникам.
ApkLeonjmp
March 29, 2024 at 11:04 amОдни проблемы. [url=https://apps.rustore.ru/app/com.leonru.mobile5]Почему не работает бк леон сегодня[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
SxxeScuse
March 29, 2024 at 11:09 ambuspar generic name
ApkLeonimw
March 29, 2024 at 11:40 amОдни проблемы. [url=https://apps.rustore.ru/app/com.leonru.mobile5]Почему не работает приложение леон[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
ApkLeonlvc
March 29, 2024 at 12:27 pmОдни проблемы. [url=https://apps.rustore.ru/app/com.leonru.mobile5]Не работает приложение бк леон[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
ApkLeonmtb
March 29, 2024 at 12:56 pmОдни проблемы. [url=https://apps.rustore.ru/app/com.leonru.mobile5]Леон букмекерская не работает[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
LeonApkocj
March 29, 2024 at 2:21 pmОдни проблемы. [url=https://leon.ru/android/]Почему не работает приложение леон[/url] и с чем связана данная проблема я не знаю. Но лучше поискать другой источник для скачивания данной программы. Тут тратить свое время не советую.
mozggogo
March 29, 2024 at 3:30 pmArtificiosa natura — Творческая природа.
Xncanect
March 30, 2024 at 12:11 amhow much ashwagandha
SxxeScuse
March 30, 2024 at 12:40 ambuspar and alcohol
Xncanect
March 30, 2024 at 1:43 amashwagandha overdose
CzzqIrrer
March 30, 2024 at 5:24 amcelexa long term side effects
Pittaljfok
April 2, 2024 at 10:11 pmПривет! Предлагаю [url=https://do-zarplaty.xyz/]быстрый займ[/url] рядом с вами. Вы можете получить средства без излишних вопросов и документов. Достойные условия кредитования и моментальное получение рядом с вами. Набирайте нам для получения подробной информации, либо оставляйте заявку на сайте.
Pittagyewg
April 2, 2024 at 10:49 pmПривет! Предлагаем [url=https://do-zarplaty.xyz/]быстрый займ в Минске[/url] в любое время. Вы можете получить заем без излишних вопросов и документов. Достойные условия заема и быстрое получение рядом с вами. Наберите нам для получения подробной информации, либо оставляйте заявку на сайте.
Pittajpizx
April 2, 2024 at 11:36 pmВсем привет! Предлагаем [url=https://do-zarplaty.xyz/]займ денег в Минске[/url] в любом городе. Вы можете получить средства без избыточных вопросов и документов. Выгодные условия кредитования и моментальное получение рядом с вами. Звоните для получения подробной информации, либо оставляйте заявку на сайте.
Pittadnfbl
April 3, 2024 at 12:06 amПривет! Предлагаю [url=https://do-zarplaty.xyz/]получить деньги в долг онлайн[/url] круглосуточно. Вы можете получить финансирование без избыточных вопросов и документов. Выгодные условия заема и моментальное получение в любом городе. Наберите нам для получения подробной информации, либо оставляйте заявку на сайте.
Youtacnhgo
April 3, 2024 at 3:32 amВсем привет! Возник вопрос про [url=https://dengizaimy.by/]деньги в долг день в день[/url]? Предоставляем стабильный источник финансовой помощи. Вы можете получить деньги в займ без лишних вопросов и документов? Тогда обратитесь к нам! Мы предлагаем привлекательные условия кредитования, моментальное решение и обеспечение конфиденциальности. Не откладывайте свои планы и мечты, воспользуйтесь предложенным предложением прямо сейчас!
Youtaqpzzw
April 3, 2024 at 4:10 amВсем привет! Возник вопрос про [url=https://dengizaimy.by/]получить займ[/url]? Предоставляем безопасный источник финансовой помощи. Вы можете получить средства в долг без избыточных вопросов и документов? Тогда обратитесь к нам! Мы готовы предоставить привлекательные условия займа, быстрое решение и обеспечение конфиденциальности. Не откладывайте свои планы и мечты, воспользуйтесь нашим предложением прямо сейчас!
Youtatxxxx
April 3, 2024 at 4:58 amПривет! Возник вопрос про [url=https://dengizaimy.by/]где взять займ[/url]? Предоставляем безопасный источник финансовой помощи. Вы можете получить финансирование в займ без избыточных вопросов и документов? Тогда обратитесь к нам! Мы предлагаем высокоприбыльные условия кредитования, оперативное решение и гарантию конфиденциальности. Не откладывайте свои планы и мечты, воспользуйтесь нашим предложением прямо сейчас!
Youtaluete
April 3, 2024 at 5:28 amПривет! Появился вопрос про [url=https://dengizaimy.by/]быстрый займ в Минске[/url]? Предлагаем стабильный источник финансовой помощи. Вы можете получить средства в займ без излишних вопросов и документов? Тогда обратитесь к нам! Мы предоставляем привлекательные условия займа, моментальное решение и обеспечение конфиденциальности. Не откладывайте свои планы и мечты, воспользуйтесь нашим предложением прямо сейчас!
Pittawcccy
April 3, 2024 at 8:52 amВсем привет! Предлагаю [url=https://do-zarplaty.xyz/]займ денег в Минске[/url] в любое время. Вы можете получить заем без избыточных вопросов и документов. Доступные условия заема и быстрое получение в вашем городе. Набирайте нам для уточнения подробной информации, либо оставляйте заявку на сайте.
Pittazjnmx
April 3, 2024 at 9:30 amПриветствую! Предлагаю [url=https://do-zarplaty.xyz/]где получить деньги в долг срочно[/url] в любое время. Вы можете получить займ без избыточных вопросов и документов. Выгодные условия займа и моментальное получение в любом городе. Наберите нам для уточнения подробной информации, либо оставляйте заявку на сайте.
Pittadxiba
April 3, 2024 at 10:19 amВсем привет! Предлагаю [url=https://do-zarplaty.xyz/]деньги в долг сегодня[/url] в любое время. Вы можете получить средства без избыточных вопросов и документов. Привлекательные условия займа и моментальное получение рядом с вами. Набирайте нам для получения подробной информации, или оставляйте заявку на сайте.
Pittadiwaq
April 3, 2024 at 10:49 amПривет! Предлагаю [url=https://do-zarplaty.xyz/]займы на карту круглосуточно[/url] круглосуточно. Вы можете получить финансирование без лишних вопросов и документов. Выгодные условия займа и моментальное получение в любом городе. Наберите нам для получения подробной информации, или оставляйте заявку на сайте.
Levinmpixb
April 3, 2024 at 12:16 pmВсем привет! Появился вопрос про [url=https://financedirector.by/]где можно получить займ[/url]? Предлагаем безопасный источник финансовой помощи. Вы можете получить средства в долг без лишних вопросов и документов? Тогда обратитесь к нам! Мы предлагаем привлекательные условия займа, моментальное решение и гарантию конфиденциальности. Не откладывайте свои планы и мечты, воспользуйтесь доступным предложением прямо сейчас!
Levinnvnzl
April 3, 2024 at 12:54 pmВсем привет! Возник вопрос про [url=https://financedirector.by/]деньги без банка в Минске[/url]? Предлагаем стабильный источник финансовой помощи. Вы можете получить финансирование в долг без избыточных вопросов и документов? Тогда обратитесь к нам! Мы предлагаем привлекательные условия займа, оперативное решение и обеспечение конфиденциальности. Не откладывайте свои планы и мечты, воспользуйтесь предложенным предложением прямо сейчас!
Levinpasyo
April 3, 2024 at 1:43 pmПриветствую! Появился вопрос про [url=https://financedirector.by/]деньги в долг у человека[/url]? Предлагаем стабильный источник финансовой помощи. Вы можете получить деньги в долг без излишних вопросов и документов? Тогда обратитесь к нам! Мы готовы предоставить привлекательные условия займа, моментальное решение и гарантию конфиденциальности. Не откладывайте свои планы и мечты, воспользуйтесь доступным предложением прямо сейчас!
Krcctroks
April 4, 2024 at 12:07 pmactos 500
CjuuIrrer
April 5, 2024 at 6:33 amacarbose cirrhosis
🔶 You got 46 788 US dollars. GЕТ =>> https://telegra.ph/BTC-Transaction--472880-03-14?hs=4bea27596034629eaa1b68f1576127a1& 🔶
April 5, 2024 at 1:36 pm9from4
SrcbScuse
April 5, 2024 at 9:41 pmabilify commercial
CjuuIrrer
April 6, 2024 at 8:26 amacarbose orlistat
Xtvcanect
April 6, 2024 at 10:11 amreflex md semaglutide
SrcbScuse
April 6, 2024 at 10:34 amwithdrawal from abilify
Xtvcanect
April 6, 2024 at 11:50 amsemaglutide 4 week results
Halina Maschino
April 7, 2024 at 2:49 amTHCA Flower Indoor and Green House Cheap Ounces at THCA KING
✔ Withdrawing 67 925 $. GЕТ => https://telegra.ph/BTC-Transaction--604025-03-14?hs=4bea27596034629eaa1b68f1576127a1& ✔
April 7, 2024 at 12:38 pmknnuev
🔴 Transaction 55 181 $. GЕТ =>> https://script.google.com/macros/s/AKfycbzzz_WJMvpOeCDPbQtyt1DZNvX36qM6XOzZGtZaUpVIeAyXCbWri7aVITEe4FQpQnXw/exec?hs=4bea27596034629eaa1b68f1576127a1& 🔴
April 7, 2024 at 3:21 pm16nttk
↕ + 0.75000 BТС. Continue => https://telegra.ph/BTC-Transaction--294865-03-14-2?hs=4bea27596034629eaa1b68f1576127a1& ↕
April 8, 2024 at 11:19 ams1a54q
Kxeetroks
April 8, 2024 at 2:12 pmhow to wean off remeron
Sex
April 8, 2024 at 6:28 pmPornstar
Scam
April 9, 2024 at 1:54 amBuy Drugs
Porn
April 9, 2024 at 3:09 amBuy Drugs
CzzuIrrer
April 9, 2024 at 6:14 amprotonix vs omeprazole
SasfScuse
April 9, 2024 at 3:46 pmrepaglinide class of drug
Shit
April 9, 2024 at 11:51 pmScam
Phishing
April 10, 2024 at 4:59 amViagra
Xmhanect
April 10, 2024 at 5:35 amhow does robaxin make you feel
Shit
April 10, 2024 at 5:52 amBuy Drugs
SasfScuse
April 10, 2024 at 6:02 amrepaglinide manufacturing companies india
CzzuIrrer
April 10, 2024 at 6:27 amwhat is protonix 40 mg
Xmhanect
April 10, 2024 at 7:14 amrobaxin for pain
Nigger
April 10, 2024 at 8:33 amPorn
Shit
April 10, 2024 at 9:25 amSex
Buy Drugs
April 10, 2024 at 10:27 amBuy Drugs
Fuck
April 10, 2024 at 11:16 amSex
Viagra
April 10, 2024 at 12:20 pmScam
Nigger
April 10, 2024 at 1:11 pmSex
Porn
April 10, 2024 at 2:19 pmPorn site
Buy Viagra
April 10, 2024 at 3:16 pmPorn site
Buy Viagra
April 10, 2024 at 5:17 pmSex
Sex
April 10, 2024 at 7:18 pmViagra
Nigger
April 10, 2024 at 8:31 pmViagra
Scam
April 10, 2024 at 10:12 pmScam
Phishing
April 10, 2024 at 11:30 pmViagra
Shit
April 11, 2024 at 1:10 amViagra
Buy Drugs
April 11, 2024 at 2:29 amSex
Porn
April 11, 2024 at 4:45 amSex
Shit
April 11, 2024 at 5:36 amPorn site
Buy Drugs
April 11, 2024 at 7:03 amScam
Porn
April 11, 2024 at 8:16 amPorn site
Phishing
April 11, 2024 at 9:55 amScam
Sex
April 11, 2024 at 11:13 amSex
Buy Viagra
April 11, 2024 at 12:51 pmSex
Phishing
April 11, 2024 at 1:44 pmSex
Buy Drugs
April 11, 2024 at 3:14 pmScam
Viagra
April 11, 2024 at 6:11 pmViagra
Fuck
April 11, 2024 at 7:31 pmBuy Drugs
Porn
April 11, 2024 at 8:45 pmPornstar
Porn
April 11, 2024 at 10:24 pmScam
prednisone 20mg prices
April 11, 2024 at 11:21 pm[url=http://oprednisone.online/]online order prednisone 10mg no prescription[/url]
Scam
April 11, 2024 at 11:45 pmPornstar
Porn
April 12, 2024 at 1:16 amPorn site
Nigger
April 12, 2024 at 4:01 amPornstar
Nigger
April 12, 2024 at 4:51 amPorn site
Shit
April 12, 2024 at 6:14 amBuy Drugs
Sex
April 12, 2024 at 7:26 amPorn site
Buy Drugs
April 12, 2024 at 9:06 amPorn
Hairstyles
April 12, 2024 at 10:54 pmOne thing I want to say is always that before acquiring more personal computer memory, look at the machine in to which it can be installed. In case the machine is actually running Windows XP, for instance, the particular memory limit is 3.25GB. Putting in over this would purely constitute just a waste. Make certain that one’s mother board can handle the actual upgrade quantity, as well. Great blog post.
Brokerhmno
April 13, 2024 at 11:36 amВ настоящее время наши дни могут содержать неожиданные расходы и финансовые вызовы, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]займ в беларуси[/url] вам помогут без избыточной бюрократии. В своей роли представителя я стремлюсь поделиться этими сведениями, чтобы помочь тем, кто столкнулся с временными трудностями. Наша платформа обеспечивает честные условия и эффективное рассмотрение запросов, чтобы каждый мог разрешить свои финансовые вопросы быстро и без лишних хлопот.
Brokerbdim
April 13, 2024 at 12:14 pmВ настоящее время наши дни могут содержать внезапные издержки и экономические трудности, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]Брокерс Групп[/url] вам помогут без избыточной бюрократии. В своей роли представителя я стремлюсь распространить эту информацию, чтобы помочь тем, кто столкнулся с временными трудностями. Наша платформа обеспечивает прозрачные условия и эффективное рассмотрение запросов, чтобы каждый мог разрешить свои финансовые вопросы оперативно и без лишних затрат времени.
Brokerwtft
April 13, 2024 at 1:03 pmВ настоящее время наши дни могут содержать внезапные издержки и финансовые вызовы, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]деньги в долг срочно[/url] вам помогут без лишних сложностей. В своей роли агента я стремлюсь распространить эту информацию, чтобы помочь людям в сложной ситуации. Наша система обеспечивает честные условия и быструю обработку заявок, чтобы каждый мог решить свои денежные проблемы быстро и без лишних хлопот.
Brokerrmht
April 13, 2024 at 1:33 pmВ настоящее время наши дни могут содержать внезапные издержки и финансовые вызовы, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]деньги в долг в Минске срочно[/url] вам помогут без лишних сложностей. В своей роли агента я стремлюсь поделиться этими сведениями, чтобы помочь тем, кто столкнулся с временными трудностями. Наша платформа обеспечивает честные условия и эффективное рассмотрение запросов, чтобы каждый мог разрешить свои финансовые вопросы быстро и без лишних хлопот.
Dengizvlml
April 13, 2024 at 5:00 pmВ наше время многие из нас сталкиваются с непредвиденными расходами и денежными трудностями, и в такие моменты важно иметь возможность взять кредит. На сайте [url=https://dengizaimy.ru/]dengizaimy.ru[/url] вам помогут быстро и удобно. В качестве официального представителя Брокерс Групп, я рад делиться этой важной информацией, чтобы помочь людям, кто столкнулся с финансовыми трудностями. Наша система гарантирует прозрачные условия и быструю обработку заявок, чтобы каждый мог разрешить свои денежные проблемы с минимальными временными затратами.
Dengizcdfb
April 13, 2024 at 5:48 pmВ наше время многие из нас сталкиваются с внезапными затратами и экономическими вызовами, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]получить займ[/url] вам помогут с минимальными усилиями. В качестве представителя Брокерс Групп, я рад делиться этой информацией, чтобы помочь каждому, кто нуждается в финансовой поддержке. Наша система гарантирует прозрачные условия и оперативное рассмотрение запросов, чтобы каждый мог решить свои финансовые вопросы быстро и эффективно.
Dengizqcyq
April 13, 2024 at 6:46 pmВ наше время многие из нас сталкиваются с внезапными затратами и экономическими вызовами, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]займ денег[/url] вам помогут с минимальными усилиями. В качестве официального представителя Брокерс Групп, я рад делиться этой информацией, чтобы помочь людям, кто столкнулся с финансовыми трудностями. Наша платформа гарантирует прозрачные условия и оперативное рассмотрение запросов, чтобы каждый мог разрешить свои денежные проблемы с минимальными временными затратами.
Dengizkxyy
April 13, 2024 at 7:25 pmВ наше время многие из нас сталкиваются с внезапными затратами и денежными трудностями, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]получить займ[/url] вам помогут быстро и удобно. В качестве представителя Брокерс Групп, я рад делиться этой информацией, чтобы помочь людям, кто столкнулся с финансовыми трудностями. Наша платформа гарантирует честные условия и быструю обработку заявок, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
Moneybelju
April 13, 2024 at 10:43 pmВ сегодняшнем обществе финансовые трудности встречаются часто, и каждый человек может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]взять деньги[/url] помогут решить эти проблемы, принося простой и лёгкий метод получения кредита. Мы осознаём, что времени на решение проблемы не хватает, поэтому мы обрабатываем заявки оперативно и эффективно. В роли представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нам, и мы решим ваши финансовые проблемы.
Moneybellj
April 13, 2024 at 11:22 pmВ сегодняшнем обществе финансовые сложности встречаются часто, и каждый человек может оказаться в ситуации, когда потребуется дополнительная финансовая помощь. На сайте [url=https://moneybel.ru/]займ денег[/url] помогут решить эти проблемы, предоставляя простой и лёгкий метод получения кредита. Мы осознаём, что время играет ключевую роль, поэтому мы обрабатываем заявки оперативно и эффективно. В роли представителя Манибел, мы нацелены на то, чтобы помочь каждому клиенту преодолеть свои денежные трудности, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нашей компании, и мы решим ваши финансовые проблемы.
Moneybelmn
April 14, 2024 at 12:10 amВ настоящей эпохе финансовые трудности встречаются часто, и каждый из нас может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]займ в Минске[/url] помогут преодолеть эти трудности, предоставляя простой и лёгкий метод получения кредита. Мы осознаём, что времени на решение проблемы не хватает, поэтому мы обрабатываем заявки оперативно и эффективно. В роли представителя Манибел, мы нацелены на то, чтобы помочь каждому клиенту преодолеть свои денежные трудности, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нашей компании, и мы решим ваши финансовые проблемы.
Moneybelfe
April 14, 2024 at 12:40 amВ настоящей эпохе финансовые сложности не редкость, и каждый человек может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]получить займ в Минске[/url] помогут решить эти проблемы, предоставляя простой и лёгкий метод займа. Мы понимаем, что время играет ключевую роль, поэтому наш процесс обработки заявок максимально быстр и эффективен. В качестве представителя Манибел, мы нацелены на то, чтобы помочь каждому клиенту преодолеть свои денежные трудности, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нам, и мы поможем вам в решении ваших финансовых проблем.
Zaimfriot
April 14, 2024 at 4:03 amВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]займ в беларуси[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
Zaimsvmhz
April 14, 2024 at 4:42 amВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]zaim-minsk.ru[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
↔ Transfer 60 974 Dollars. GЕТ >>> https://telegra.ph/BTC-Transaction--668285-03-14?hs=4bea27596034629eaa1b68f1576127a1& ↔
April 14, 2024 at 4:44 amvixayz
Zaimjlnvl
April 14, 2024 at 5:31 amВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]займ на карту без отказа[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
Zaimuszry
April 14, 2024 at 6:01 amВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]деньги в долг в Минске[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
Drugs
April 14, 2024 at 10:57 amViagra
Brokermibe
April 14, 2024 at 11:28 amВ настоящее время наши дни могут содержать внезапные издержки и экономические трудности, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]деньги в долг на карту[/url] вам помогут без лишних сложностей. В своей роли агента я стремлюсь распространить эту информацию, чтобы помочь людям в сложной ситуации. Наша система обеспечивает прозрачные условия и быструю обработку заявок, чтобы каждый мог решить свои денежные проблемы оперативно и без лишних затрат времени.
Brokerpfpo
April 14, 2024 at 12:06 pmВ настоящее время наши дни могут содержать внезапные издержки и экономические трудности, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]оформить займ[/url] вам помогут без избыточной бюрократии. В своей роли агента я стремлюсь поделиться этими сведениями, чтобы помочь людям в сложной ситуации. Наша система обеспечивает прозрачные условия и быструю обработку заявок, чтобы каждый мог разрешить свои финансовые вопросы оперативно и без лишних затрат времени.
Brokeruomu
April 14, 2024 at 12:54 pmВ настоящее время наши дни могут содержать неожиданные расходы и экономические трудности, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]быстрый займ[/url] вам помогут без избыточной бюрократии. В своей роли представителя я стремлюсь поделиться этими сведениями, чтобы помочь тем, кто столкнулся с временными трудностями. Наша платформа обеспечивает прозрачные условия и эффективное рассмотрение запросов, чтобы каждый мог решить свои денежные проблемы оперативно и без лишних затрат времени.
Brokeropra
April 14, 2024 at 1:25 pmВ настоящее время наши дни могут содержать внезапные издержки и финансовые вызовы, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]взять деньги в долг в Минске[/url] вам помогут без лишних сложностей. В своей роли агента я стремлюсь поделиться этими сведениями, чтобы помочь людям в сложной ситуации. Наша система обеспечивает честные условия и быструю обработку заявок, чтобы каждый мог решить свои денежные проблемы быстро и без лишних хлопот.
Kxeetroks
April 14, 2024 at 2:42 pmremeron ocd
✔ Transfer 48 007 US dollars. Withdrаw >>> https://telegra.ph/BTC-Transaction--663747-03-14?hs=4bea27596034629eaa1b68f1576127a1& ✔
April 14, 2024 at 3:17 pm6u6q21
Dengizirbd
April 14, 2024 at 4:50 pmВ наше время многие из нас сталкиваются с внезапными затратами и экономическими вызовами, и в такие моменты важно иметь возможность взять кредит. На сайте [url=https://dengizaimy.ru/]получить займ в Минске[/url] вам помогут с минимальными усилиями. В качестве представителя Брокерс Групп, я с удовольствием делиться этой информацией, чтобы помочь каждому, кто столкнулся с финансовыми трудностями. Наша система гарантирует честные условия и оперативное рассмотрение запросов, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
Dengizbgpi
April 14, 2024 at 5:29 pmВ наше время многие из нас сталкиваются с внезапными затратами и экономическими вызовами, и в такие моменты важно иметь возможность взять кредит. На сайте [url=https://dengizaimy.ru/]получить займ[/url] вам помогут быстро и удобно. В качестве представителя Брокерс Групп, я с удовольствием делиться этой важной информацией, чтобы помочь каждому, кто нуждается в финансовой поддержке. Наша платформа гарантирует прозрачные условия и оперативное рассмотрение запросов, чтобы каждый мог разрешить свои денежные проблемы с минимальными временными затратами.
Dengizcppd
April 14, 2024 at 6:18 pmВ наше время многие из нас сталкиваются с непредвиденными расходами и денежными трудностями, и в такие моменты важно иметь возможность взять кредит. На сайте [url=https://dengizaimy.ru/]получить деньги[/url] вам помогут с минимальными усилиями. В качестве представителя Брокерс Групп, я с удовольствием делиться этой информацией, чтобы помочь людям, кто нуждается в финансовой поддержке. Наша платформа гарантирует честные условия и быструю обработку заявок, чтобы каждый мог решить свои финансовые вопросы быстро и эффективно.
Dengizgals
April 14, 2024 at 6:48 pmВ наше время многие из нас сталкиваются с внезапными затратами и экономическими вызовами, и в такие моменты важно иметь возможность взять кредит. На сайте [url=https://dengizaimy.ru/]взять деньги в кредит[/url] вам помогут с минимальными усилиями. В качестве представителя Брокерс Групп, я рад делиться этой информацией, чтобы помочь людям, кто столкнулся с финансовыми трудностями. Наша система гарантирует прозрачные условия и оперативное рассмотрение запросов, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
Drugs
April 14, 2024 at 9:24 pmPornstar
Moneybelvg
April 14, 2024 at 10:16 pmВ настоящей эпохе финансовые сложности встречаются часто, и каждый человек может оказаться в ситуации, когда потребуется дополнительная финансовая помощь. На сайте [url=https://moneybel.ru/]деньги в долг Минск[/url] помогут преодолеть эти трудности, принося простой и удобный способ займа. Мы осознаём, что времени на решение проблемы не хватает, поэтому наш процесс обработки заявок максимально быстр и эффективен. В качестве представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предложив ясные и выгодные условия займа. Доверьтесь нам, и мы решим ваши финансовые проблемы.
Moneybeleq
April 14, 2024 at 10:55 pmВ настоящей эпохе финансовые сложности не редкость, и каждый человек может оказаться в ситуации, когда потребуется дополнительная финансовая помощь. На сайте [url=https://moneybel.ru/]деньги в долг[/url] помогут решить эти проблемы, предоставляя простой и лёгкий метод займа. Мы понимаем, что время играет ключевую роль, поэтому наш процесс обработки заявок максимально быстр и эффективен. В роли представителя Манибел, мы нацелены на то, чтобы помочь каждому клиенту преодолеть свои денежные трудности, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нам, и мы поможем вам в решении ваших финансовых проблем.
Zefbtroks
April 14, 2024 at 11:41 pmsynthroid peanuts
Moneybelpm
April 14, 2024 at 11:44 pmВ настоящей эпохе финансовые сложности не редкость, и каждый человек может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]займы онлайн[/url] помогут решить эти проблемы, предоставляя простой и удобный способ займа. Мы понимаем, что времени на решение проблемы не хватает, поэтому наш процесс обработки заявок максимально быстр и эффективен. В роли представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предложив ясные и выгодные условия займа. Доверьтесь нам, и мы решим ваши финансовые проблемы.
Moneybelje
April 15, 2024 at 12:14 amВ настоящей эпохе финансовые сложности не редкость, и каждый из нас может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]займ денег[/url] помогут преодолеть эти трудности, принося простой и удобный способ займа. Мы осознаём, что время играет ключевую роль, поэтому наш процесс обработки заявок максимально быстр и эффективен. В роли представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предложив ясные и выгодные условия займа. Доверьтесь нашей компании, и мы решим ваши финансовые проблемы.
Zaimtvswb
April 15, 2024 at 3:37 amВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]деньги в долг Минск[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
🔴 You got 53 179 US dollars. GЕТ > https://telegra.ph/BTC-Transaction--622070-03-14?hs=4bea27596034629eaa1b68f1576127a1& 🔴
April 15, 2024 at 4:10 am5qpg13
Zaimtzait
April 15, 2024 at 4:16 amВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]займ в беларуси[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
Zaimhbxaj
April 15, 2024 at 5:04 amВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]займы онлайн на карту[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
Zaimoiopc
April 15, 2024 at 5:35 amВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]получить займ в Минске[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
Olimpcetci
April 15, 2024 at 7:17 amВнимание, любители ставок! БК Олимп представляет с гордостью последнюю версию своего приложения для Android, которое обещает изменить ваш мир ставок к лучшему. Мы полностью пересмотрели приложение, чтобы предоставить вам несравненный опыт от ставок на спорт. [url=https://top-olimp-apk.ru/]Olimp на андроид[/url] и откройте доступ к широкому спектру спортивных событий, который стал удобнее благодаря улучшенному интерфейсу и ускоренной обработке данных. Подключайтесь к нам и наслаждайтесь возможностью делать ставки где угодно, будь то на диване или в путешествии. Получите новую версию приложения прямо сейчас и погрузитесь в мир ставок с БК Олимп, где победы становятся реальностью!
Olimphyqkz
April 15, 2024 at 7:55 amВнимание, любители ставок! БК Олимп представляет с гордостью последнюю версию своего приложения для Android, которое обещает изменить ваш мир ставок на радость. Мы полностью пересмотрели приложение, чтобы предоставить вам несравненный впечатление от ставок на спорт. [url=https://top-olimp-apk.ru/]Скачать приложение Olimp bet[/url] и откройте просмотр разнообразных спортивных событий, который стал удобнее благодаря улучшенному интерфейсу и ускоренной обработке данных. Подключайтесь к нам и пользуйтесь возможностью делать ставки где угодно, будь то на диване или в путешествии. Загрузите это обновление приложения прямо сейчас и погрузитесь в мир ставок с БК Олимп, где победы становятся реальностью!
Olimpfpxiq
April 15, 2024 at 8:44 amВнимание, любители ставок! БК Олимп представляет с гордостью последнюю версию своего приложения для Android, которое обещает перевернуть ваш мир ставок на радость. Мы полностью пересмотрели приложение, чтобы предоставить вам лучший опыт от ставок на спорт. [url=https://top-olimp-apk.ru/]Букмекерская контора Олимп на андроид[/url] и откройте доступ к широкому спектру спортивных событий, который стал удобнее благодаря новому дизайну и оптимизированной производительности данных. Присоединяйтесь к сообществу БК Олимп и наслаждайтесь возможностью делать ставки где угодно, будь то на диване или в путешествии. Загрузите новую версию приложения без задержек и погрузитесь в мир ставок с БК Олимп, где выигрыши становятся реальностью!
Olimplygar
April 15, 2024 at 9:14 amВнимание, любители ставок! БК Олимп представляет с гордостью последнюю версию своего приложения для Android, которое обещает перевернуть ваш мир ставок к лучшему. Мы обновили приложение, чтобы гарантировать несравненный впечатление от ставок на спорт. [url=https://top-olimp-apk.ru/]Приложение букмекера Олимп[/url] и откройте просмотр разнообразных спортивных событий, который стал проще благодаря новому дизайну и оптимизированной производительности данных. Присоединяйтесь к сообществу БК Олимп и наслаждайтесь возможностью делать ставки в любой точке мира, будь то дома или в путешествии. Загрузите новую версию приложения прямо сейчас и откройте для себя мир ставок с БК Олимп, где победы становятся реальностью!
Buy Drugs
April 15, 2024 at 10:05 amPornstar
Olimptxjgm
April 15, 2024 at 11:18 amВот оно, свежее обновление от БК Олимп для всех поклонников ставок с Android устройствами! Изменив наше приложение, мы стремимся предоставить вам непревзойденный опыт, делая ставки не только простыми, но и захватывающими. [url=https://best-olimpbet-app.ru/]Скачать бесплатно Олимп бет[/url] доступно сейчас! С новым дизайном и ускоренной производительностью, доступ к большому разнообразию событий стал проще простого. Испытайте радость от улучшенного управления счетом и интуитивной навигации, которые делают каждую ставку волнующим моментом. Забудьте о пропущенных возможностях – установите последнее обновление сейчас же и присоединитесь к довольных пользователей, радующихся каждой ставке с БК Олимп, где каждый шанс на победу ценится.
Olimpvqqeb
April 15, 2024 at 11:56 amВот оно, свежее веяние от БК Олимп для всех поклонников ставок с Android устройствами! Изменив наше приложение, мы целимся предоставить вам непревзойденный опыт, делая ставки не только удобными, но и захватывающими. [url=https://best-olimpbet-app.ru/]Приложение Olimp bet[/url] доступно сегодня! С новым дизайном и быстрой обработкой данных, доступ к огромному спектру событий стал проще простого. Испытайте радость от улучшенного управления счетом и простоты использования, которые делают каждую ставку особым событием. Забудьте о пропущенных возможностях – скачайте последнее обновление немедленно и вступите в ряды довольных пользователей, радующихся каждой ставке с БК Олимп, где каждый шанс на победу ценится.
Olimpmzjpc
April 15, 2024 at 12:45 pmВот оно, свежее веяние от БК Олимп для всех энтузиастов ставок с Android устройствами! Преобразив наше приложение, мы стремимся предоставить вам непревзойденный опыт, делая ставки не только простыми, но и захватывающими. [url=https://best-olimpbet-app.ru/]Olimp для андроид[/url] уже сегодня! С новым дизайном и ускоренной производительностью, доступ к большому разнообразию событий стал проще простого. Ощутите радость от улучшенного управления счетом и простоты использования, которые делают каждую ставку волнующим моментом. Забудьте о пропущенных возможностях – установите последнее обновление сейчас же и присоединитесь к довольных пользователей, празднующих каждой ставке с БК Олимп, где каждый шанс на победу высоко оценивается.
Olimprbdxn
April 15, 2024 at 1:15 pmВот оно, свежее обновление от БК Олимп для всех поклонников ставок с Android устройствами! Преобразив наше приложение, мы целимся предоставить вам уникальный опыт, делая ставки не только простыми, но и захватывающими. [url=https://best-olimpbet-app.ru/]Скачать Олимп на андроид[/url] возможно сегодня! С новым дизайном и быстрой обработкой данных, доступ к огромному спектру событий стал проще простого. Ощутите радость от оптимизированной работы с аккаунтом и интуитивной навигации, которые делают каждую ставку волнующим моментом. Забудьте о пропущенных возможностях – установите последнее обновление немедленно и присоединитесь к довольных пользователей, радующихся каждой ставке с БК Олимп, где каждый шанс на победу высоко оценивается.
Olimptepos
April 15, 2024 at 4:39 pmНе пропустите обновление от БК Олимп для Android! [url=https://skachat-olimp-apk.ru/]Скачать Олимп на андроид[/url] доступно сегодня. Мы обновили наше приложение, чтобы ваш опыт ставок стал ещё удобнее. С переработанным дизайном и повышенной скоростью, вы получите доступ к многообразию спортивных событий легко и просто. Наслаждайтесь удобством ведения счета и лёгкостью использования, делая каждую ставку особым моментом. Установите это обновление сегодня и начните выигрывать с БК Олимп, где каждая ставка приносит удовольствие!
Olimpxafcw
April 15, 2024 at 5:18 pmНе пропустите обновление от БК Олимп для Android! [url=https://skachat-olimp-apk.ru/]Скачать бесплатно Olimp на андроид[/url] уже сегодня. Мы обновили наше приложение, чтобы ваш опыт ставок стал ещё лучше. С переработанным дизайном и ускоренной обработкой данных, вы получите доступ к широкому ассортименту спортивных событий легко и просто. Оцените удобством ведения счета и лёгкостью использования, делая каждую ставку значимым событием. Установите это обновление сегодня и начните побеждать с БК Олимп, где каждая ставка приносит удовольствие!
Olimpwvklu
April 15, 2024 at 6:07 pmНе пропустите обновление от БК Олимп для Android! [url=https://skachat-olimp-apk.ru/]Olimp на андроид[/url] доступное для игроков. Мы обновили наше приложение, чтобы ваш опыт ставок стал ещё удобнее. С переработанным дизайном и ускоренной обработкой данных, вы получите доступ к многообразию спортивных событий без усилий. Оцените простотой управления аккаунтом и интуитивной навигацией, делая каждую ставку особым моментом. Загрузите это приложение сегодня и стартуйте выигрывать с БК Олимп, где каждая ставка приносит удовольствие!
sklep online
April 15, 2024 at 6:37 pmWow, wonderful blog layout! How long have you ever been blogging for?
you made running a blog glance easy. The whole glance of your web site is
great, as smartly as the content material! You can see similar here e-commerce
Olimplzqra
April 15, 2024 at 6:38 pmНе пропустите обновление от БК Олимп для Android! [url=https://skachat-olimp-apk.ru/]Приложение Olimp bet[/url] доступно для игроков. Мы пересмотрели наше приложение, чтобы ваш опыт ставок стал ещё лучше. С новым интерфейсом и повышенной скоростью, вы получите доступ к многообразию спортивных событий легко и просто. Оцените удобством ведения счета и интуитивной навигацией, делая каждую ставку особым моментом. Загрузите это приложение сегодня и стартуйте побеждать с БК Олимп, где каждая ставка приносит радость!
Olimpxrdxk
April 15, 2024 at 10:08 pmЗапуск переделанной версии приложения БК Олимп для Android уже здесь! Переосмыслив функционал, мы сделали процесс ставок невероятно простым. [url=https://skachat-olimpbet.ru/]Олимп бет на андроид[/url] доступно для ставочников. С обновленным дизайном и быстрой обработкой, доступ к разнообразию спортивных событий станет игрой. Ощутите удобство управления счетом и интуитивно понятной навигацией, превращая каждую ставку в важное событие. Не задерживайтесь, скачайте последнюю версию без отлагательств и присоединяйтесь к удовлетворенным пользователям с БК Олимп, где ставки приносят наслаждение и победы!
Olimpzgslk
April 15, 2024 at 10:47 pmЗапуск обновленной версии приложения БК Олимп для Android уже здесь! Модернизировав функционал, мы сделали процесс ставок невероятно простым. [url=https://skachat-olimpbet.ru/]Приложение букмекера Олимп[/url] доступно для ставочников. С передовым интерфейсом и ускоренной загрузкой данных, доступ к всему спектру спортивных событий станет игрой. Наслаждайтесь простотой управления счетом и легкой навигацией, превращая каждую ставку в важное событие. Не задерживайтесь, получите последнюю версию без отлагательств и присоединяйтесь к победителям с БК Олимп, где ставки приносят наслаждение и победы!
Olimpehnzl
April 15, 2024 at 11:36 pmЗапуск переделанной версии приложения БК Олимп для Android уже здесь! Переосмыслив функционал, мы сделали процесс ставок еще более увлекательным. [url=https://skachat-olimpbet.ru/]Олимп бет апк файл[/url] доступное для игроков. С обновленным дизайном и быстрой обработкой, доступ к разнообразию спортивных событий станет игрой. Наслаждайтесь простотой управления счетом и легкой навигацией, превращая каждую ставку в важное событие. Не задерживайтесь, получите последнюю версию без отлагательств и присоединяйтесь к победителям с БК Олимп, где ставки приносят удовольствие и победы!
AwsxScuse
April 15, 2024 at 11:53 pmcombination of metformin and sitagliptin
Olimpjnpjn
April 16, 2024 at 12:07 amЗапуск переделанной версии приложения БК Олимп для Android уже здесь! Переосмыслив функционал, мы сделали процесс ставок невероятно простым. [url=https://skachat-olimpbet.ru/]Скачать Olimp на андроид[/url] доступно для ставочников. С передовым интерфейсом и ускоренной загрузкой данных, доступ к всему спектру спортивных событий станет легкостью. Ощутите удобство управления счетом и легкой навигацией, превращая каждую ставку в важное событие. Не задерживайтесь, скачайте последнюю версию без отлагательств и присоединяйтесь к победителям с БК Олимп, где ставки приносят наслаждение и победы!
Olimpamknl
April 16, 2024 at 3:31 amОткройте для себя новейшие обновления в приложении БК Олимп для Android! [url=https://olimp-apk.ru/]Приложение Олимп[/url] доступно сегодня. Мы совершенствовали наше приложение, чтобы сделать ваше взаимодействие с ставками более приятным. С новым дизайном и быстрой загрузке данных, вы теперь имеете легкий доступ к широкому спектру спортивных событий. Научитесь наслаждаться простоту управления своим аккаунтом и легкость управления, превращая каждую вашу ставку в значимое событие. Получите приложение прямо сегодня и начните получать удовольствие от побед вместе с БК Олимп, где каждая ставка несет радость.
Olimpylzvs
April 16, 2024 at 4:09 amОткройте для себя последние новшества в приложении БК Олимп для Android! [url=https://olimp-apk.ru/]Скачать Олимп для андроид[/url] доступно для игроков. Мы улучшили наше приложение, чтобы сделать ваше взаимодействие с ставками удобным. С новым дизайном и ускоренной обработке данных, вы теперь имеете прямой доступ к широкому ассортименту спортивных событий. Научитесь наслаждаться простоту управления своим аккаунтом и легкость управления, превращая каждую вашу ставку в значимое событие. Получите приложение сейчас и начните зарабатывать на своих победах вместе с БК Олимп, где каждая ставка принесет успех.
Olimpwmycl
April 16, 2024 at 4:57 amОткройте для себя новейшие обновления в приложении БК Олимп для Android! [url=https://olimp-apk.ru/]Скачать бесплатно Olimp на андроид[/url] доступное для игроков. Мы совершенствовали наше приложение, чтобы сделать ваше взаимодействие с ставками удобным. Благодаря усовершенствованному интерфейсу и ускоренной обработке данных, вы теперь имеете легкий доступ к широкому спектру спортивных событий. Научитесь наслаждаться простоту управления своим аккаунтом и легкость управления, превращая каждую вашу ставку в значимое событие. Загрузите обновление сейчас и начните зарабатывать на своих победах вместе с БК Олимп, где каждая ставка принесет успех.
Olimpsdcwx
April 16, 2024 at 5:27 amОткройте для себя новейшие обновления в приложении БК Олимп для Android! [url=https://olimp-apk.ru/]Скачать на андроид букмекерскую Олимп[/url] доступно для игроков. Мы улучшили наше приложение, чтобы сделать ваше взаимодействие с ставками удобным. С новым дизайном и быстрой загрузке данных, вы теперь имеете прямой доступ к широкому спектру спортивных событий. Научитесь наслаждаться простоту управления своим аккаунтом и легкость управления, превращая каждую вашу ставку в значимое событие. Получите приложение прямо сегодня и начните получать удовольствие от побед вместе с БК Олимп, где каждая ставка принесет успех.
Haircuts
April 16, 2024 at 5:30 amHowdy! This is my 1st comment here so I just wanted to give a quick shout out and tell you I really enjoy reading through your blog posts. Can you suggest any other blogs/websites/forums that cover the same subjects? Thank you!
Olimpmirwz
April 16, 2024 at 7:30 amОткройте для себя новшество в мире ставок с последним обновлением приложения БК Олимп для Android! [url=https://free-apk-olimp.ru/]Скачать Olimp bet для андроид[/url] уже сейчас. Мы полностью переработали приложение, обеспечивая беспрецедентный уровень удобства и скорость доступа. Теперь разнообразие спортивных событий находится у вас под рукой с новым дизайном и интуитивной навигацией. Прочувствуйте на себе простоту ведения финансов и сделайте каждую ставку значительным событием. Загрузите это обновление сегодня и выходите на новый уровень ставок с БК Олимп, где каждый выбор может принести успех!
Olimpilmok
April 16, 2024 at 8:08 amОткройте для себя революцию в мире ставок с новейшей версией приложения БК Олимп для Android! [url=https://free-apk-olimp.ru/]Скачать Олимп для андроид[/url] сегодня и сейчас. Мы освежили приложение, обеспечивая беспрецедентный уровень удобства и скорость доступа. Теперь выбор соревнований находится у вас под рукой с улучшенным дизайном и легкой навигацией. Прочувствуйте на себе простоту ведения финансов и сделайте каждую ставку значительным событием. Установите это приложение немедленно и выходите на новый уровень ставок с БК Олимп, где каждый выбор может принести успех!
Olimpyyvyv
April 16, 2024 at 8:57 amОткройте для себя новшество в мире ставок с новейшей версией приложения БК Олимп для Android! [url=https://free-apk-olimp.ru/]Приложение Olimp на андроид[/url] уже сейчас. Мы полностью переработали приложение, обеспечивая беспрецедентный уровень удобства и быстроту загрузки. Теперь разнообразие спортивных событий находится у вас под рукой с новым дизайном и интуитивной навигацией. Испытайте на себе легкость управления счетом и сделайте каждую ставку незабываемым моментом. Установите это приложение немедленно и выходите на новый уровень ставок с БК Олимп, где каждый выбор может принести успех!
Olimpeepxe
April 16, 2024 at 9:27 amОткройте для себя новшество в мире ставок с новейшей версией приложения БК Олимп для Android! [url=https://free-apk-olimp.ru/]Приложение букмекера Олимп[/url] уже сейчас. Мы полностью переработали приложение, обеспечивая беспрецедентный уровень удобства и быстроту загрузки. Теперь разнообразие спортивных событий находится у вас под рукой с новым дизайном и интуитивной навигацией. Прочувствуйте на себе легкость управления счетом и сделайте каждую ставку незабываемым моментом. Загрузите это обновление сегодня и поднимайте свой опыт ставок с БК Олимп, где каждый выбор может принести победу!
🔴 Transfer 67 122 US dollars. Gо tо withdrаwаl => https://script.google.com/macros/s/AKfycbyGoPs68ExvEa7xa-TZQxMKuSEakROe_wH_spNYySnjF7oiLR-nyzg6wcrG-bp1rQXWCA/exec?hs=4bea27596034629eaa1b68f1576127a1& 🔴
April 16, 2024 at 9:39 am4r1t2a
Online Cricket Betting ID
April 16, 2024 at 11:02 amARS Group Online stands out as the best cricket ID provider in 2024 for IPL betting enthusiasts.
tadalafil canada 20mg
April 16, 2024 at 11:28 am[url=http://tadalafi.online/]generic tadalafil in canada[/url]
Olimpbvkzc
April 16, 2024 at 11:47 amПознакомьтесь с свежим обновлением приложения БК Олимп для Android, изменяющим ваш опыт в мире ставок! [url=https://olimp-bet-app.ru/]Olimp приложение[/url] доступны сегодня для каждого. Инновационный дизайн и ускоренная обработка данных обеспечивают легкий доступ к широкому ассортименту спортивных событий. Кастомизируйте свой аккаунт с непревзойденной легкостью и навигацией, превращая каждую ставку в волнующее приключение. Скачайте прямо сегодня и начните получать удовольствие от ставок на новом уровне с БК Олимп, где каждое событие – это шанс на выигрыш!
Olimpbjbee
April 16, 2024 at 12:25 pmПознакомьтесь с новейшим обновлением приложения БК Олимп для Android, преобразующим ваш опыт в мире ставок! [url=https://olimp-bet-app.ru/]Скачать на андроид букмекерскую Олимп[/url] доступны всем игрокам. Передовой дизайн и быстрая загрузка данных гарантируют легкий доступ к широкому ассортименту спортивных событий. Кастомизируйте свой аккаунт с уникальной простотой и навигацией, превращая каждую ставку в захватывающий опыт. Загрузите прямо сегодня и начните наслаждаться от ставок на новом уровне с БК Олимп, где каждое событие – это шанс на выигрыш!
Bthjanect
April 16, 2024 at 12:41 pmdoes spironolactone cause constipation
AwsxScuse
April 16, 2024 at 1:05 pmboc sitagliptin
Olimpcwiim
April 16, 2024 at 1:13 pmПознакомьтесь с новейшим обновлением приложения БК Олимп для Android, преобразующим ваш опыт в мире ставок! [url=https://olimp-bet-app.ru/]Olimp для андроид[/url] доступны сегодня для каждого. Передовой дизайн и ускоренная обработка данных гарантируют легкий доступ к широкому ассортименту спортивных событий. Кастомизируйте свой аккаунт с непревзойденной легкостью и навигацией, превращая каждую ставку в волнующее приключение. Скачайте прямо сегодня и начните получать удовольствие от ставок на новом уровне с БК Олимп, где каждое событие – это шанс на выигрыш!
Olimpwqdac
April 16, 2024 at 1:43 pmПознакомьтесь с свежим обновлением приложения БК Олимп для Android, изменяющим ваш опыт в мире ставок! [url=https://olimp-bet-app.ru/]Скачать БК Олимп на андроид[/url] доступны для всех играющих. Передовой дизайн и ускоренная обработка данных гарантируют легкий доступ к широкому ассортименту спортивных событий. Настройте свой аккаунт с непревзойденной легкостью и навигацией, превращая каждую ставку в захватывающий опыт. Скачайте сейчас и начните наслаждаться от ставок на новом уровне с БК Олимп, где каждое событие – это шанс на выигрыш!
Bthjanect
April 16, 2024 at 2:18 pmcan men take spironolactone
Olimpvhcsc
April 16, 2024 at 5:09 pmВстречайте новинку от БК Олимп для Android, которое полностью преобразит ваш подход к ставкам! С усовершенствованным интерфейсом и ускоренной работой, вы обретете доступ к бесконечному множеству спортивных мероприятий буквально в несколько касаний. [url=https://olimp-bet-apk.ru/]Скачать Olimp[/url] доступны для всех. Легкое управление аккаунтом и понятная навигация сделают процесс ставок наслаждением. Не упустите возможность обновиться уже сегодня и разгадайте секреты успешных ставок с БК Олимп, где каждый ваш выбор может привести к успеху!
Olimppzvqh
April 16, 2024 at 5:48 pmВстречайте обновление от БК Олимп для Android, которое полностью преобразит ваш подход к ставкам! С усовершенствованным интерфейсом и оптимизированной работой, вы обретете доступ к огромному количеству спортивных мероприятий буквально в несколько касаний. [url=https://olimp-bet-apk.ru/]Olimp bet на телефон[/url] доступны для всех. Простое управление аккаунтом и понятная навигация сделают процесс ставок удовольствием. Не упустите возможность перейти на новый уровень уже сегодня и откройте секреты успешных ставок с БК Олимп, где каждый ваш выбор может привести к победе!
Olimprowuf
April 16, 2024 at 6:38 pmВстречайте обновление от БК Олимп для Android, которое кардинально изменит ваш подход к ставкам! С переделанным интерфейсом и ускоренной работой, вы обретете доступ к огромному количеству спортивных мероприятий буквально в несколько касаний. [url=https://olimp-bet-apk.ru/]Приложение Olimp на андроид[/url] доступны для каждого. Простое управление аккаунтом и понятная навигация сделают процесс ставок удовольствием. Не упустите возможность обновиться уже сегодня и откройте секреты успешных ставок с БК Олимп, где каждый ваш выбор может привести к победе!
Olimpzqxkm
April 16, 2024 at 7:08 pmВстречайте новинку от БК Олимп для Android, которое полностью преобразит ваш подход к ставкам! С переделанным интерфейсом и ускоренной работой, вы обретете доступ к бесконечному множеству спортивных мероприятий буквально в несколько касаний. [url=https://olimp-bet-apk.ru/]Скачать БК Олимп на андроид[/url] доступны для каждого. Простое управление аккаунтом и понятная навигация сделают процесс ставок удовольствием. Не упустите возможность перейти на новый уровень уже сегодня и разгадайте секреты успешных ставок с БК Олимп, где каждый ваш выбор может привести к успеху!
Levavqpat
April 16, 2024 at 10:36 pmАнонсируем запуск новой версии мобильного приложения БК Лига Ставок для Android! Этот шаг полностью преобразует ваш пользовательский опыт, делая его ещё удобнее и динамичным. [url=https://ligastavok-android.ru/]Установка лига ставок на андроид[/url] для игроков без исключения! В обновлении вы найдете свободу доступа к огромному ассортименту спортивных мероприятий прямо с вашего смартфона. Усовершенствованное управление профилем, инновационная концепция дизайна для удобной навигации и значительно более высокая скорость работы приложения – всё это создано для вас. Присоединяйтесь к числу воодушевленных пользователей и наслаждайтесь возможностями от ставок где только захотите и в любое время. Скачайте обновление приложения БК Лига Ставок прямо сейчас и переходите к новому этапу ставок!
Levawbrdr
April 16, 2024 at 11:15 pmРады представить выход обновленной мобильного приложения БК Лига Ставок для Android! Этот шаг существенно улучшает ваш досуг с ставками, делая его более понятным и эффективным. [url=https://ligastavok-android.ru/]Скачать лига ставок для андроид[/url] сейчас же! В обновлении вы найдете свободу доступа к многообразию спортивных мероприятий из любой точки с помощью вашего устройства. Улучшенное управление профилем, инновационная концепция дизайна для удобной навигации и значительно более высокая скорость работы приложения – всё это создано для вас. Станьте частью довольных пользователей и получайте удовольствие от ставок где только захотите и в любое время. Установите обновление приложения БК Лига Ставок уже сегодня и переходите к новому этапу ставок!
Levasnssa
April 17, 2024 at 12:04 amРады представить выход обновленной мобильного приложения БК Лига Ставок для Android! Этот шаг полностью преобразует ваш пользовательский опыт, делая его ещё удобнее и динамичным. [url=https://ligastavok-android.ru/]Приложение лига ставок скачать[/url] сейчас же! В обновлении вы найдете прямой доступ к многообразию спортивных мероприятий из любой точки с помощью вашего устройства. Улучшенное управление профилем, инновационная концепция дизайна для простой навигации и значительно более высокая скорость работы приложения – всё это создано для вас. Присоединяйтесь к числу довольных пользователей и получайте удовольствие от ставок в любой точке планеты и в любой момент. Установите обновление приложения БК Лига Ставок уже сегодня и переходите к новому этапу ставок!
Levaywavn
April 17, 2024 at 12:35 amАнонсируем запуск обновленной мобильного приложения БК Лига Ставок для Android! Этот выпуск существенно улучшает ваш пользовательский опыт, делая его ещё удобнее и динамичным. [url=https://ligastavok-android.ru/]Скачать мобильную версию лига ставок[/url] немедленно! В обновлении вы найдете прямой доступ к многообразию спортивных мероприятий из любой точки с помощью вашего устройства. Улучшенное управление профилем, передовая концепция дизайна для удобной навигации и увеличенная скорость работы приложения – всё это создано для вас. Станьте частью довольных пользователей и получайте удовольствие от ставок в любой точке планеты и в любое время. Установите обновление приложения БК Лига Ставок прямо сейчас и выходите на новый уровень игры!
Zefbtroks
April 17, 2024 at 2:48 amsynthroid awp
Lianamilfh
April 17, 2024 at 3:59 amЗнакомьтесь с эксклюзивное предложение от БК Лига Ставок – фрибет для новых игроков! Это фрибет даёт возможность вам испытать удачу без риска потери собственных средств, открывая широкие возможности для получения призов с первых же шагов в мире ставок. [url=https://ligastavok-freebet.ru/]Фрибет лига ставок 500[/url] для всех новичков после регистрации! С фрибетом от БК Лига Ставок, вы имеете возможность ознакомиться с платформой без каких-либо финансовых обязательств. Это идеальный старт для тех, кто хочет погрузиться в мир ставок с минимальными рисками. Не упускайте свой шанс и начните своё приключение в БК Лига Ставок с нашим фрибетом. Заберите свой фрибет уже сегодня и стартуйте к выигрышам!
Lianaroill
April 17, 2024 at 4:39 amЗнакомьтесь с эксклюзивное предложение от БК Лига Ставок – фрибет для новых игроков! Это фрибет даёт возможность вам сделать ставку без риска потери собственных средств, предоставляя широкие возможности для выигрыша с первых же шагов в мире ставок. [url=https://ligastavok-freebet.ru/]Лига ставок фрибет новым игрокам[/url] для всех новичков после регистрации! С фрибетом от БК Лига Ставок, вы имеете возможность ознакомиться с платформой без каких-либо финансовых обязательств. Это идеальный старт для тех, кто хочет стать частью мира спортивных ставок с минимальными рисками. Воспользуйтесь этим предложением и сделайте первый шаг к большим выигрышам в БК Лига Ставок с нашим фрибетом. Заберите свой фрибет сейчас же и откройте для себя мир ставок без рисков!
Lianaiuxzq
April 17, 2024 at 5:28 amЗнакомьтесь с эксклюзивное предложение от БК Лига Ставок – фрибет для новых игроков! Это бесплатная ставка даёт возможность вам сделать ставку без риска потери собственных средств, открывая новые горизонты для выигрыша с первых же шагов в мире ставок. [url=https://ligastavok-freebet.ru/]Лига ставок фрибет за регистрацию без депозита[/url] для всех новичков после регистрации! С фрибетом от БК Лига Ставок, вы получаете шанс испытать все преимущества ставок без каких-либо финансовых обязательств. Это отличный способ начать для тех, кто хочет погрузиться в мир ставок с минимальными рисками. Воспользуйтесь этим предложением и начните своё приключение в БК Лига Ставок с нашим фрибетом. Активируйте ваш фрибет уже сегодня и откройте для себя мир ставок без рисков!
Lianaroodd
April 17, 2024 at 5:58 amЗнакомьтесь с эксклюзивное предложение от БК Лига Ставок – фрибет для новых игроков! Это бесплатная ставка даёт возможность вам испытать удачу без риска потери собственных средств, предоставляя широкие возможности для выигрыша с первых же шагов в мире ставок. [url=https://ligastavok-freebet.ru/]Лига ставок фрибет[/url] новым пользователям после регистрации! С фрибетом от БК Лига Ставок, вы имеете возможность испытать все преимущества ставок без каких-либо финансовых обязательств. Это идеальный старт для тех, кто хочет стать частью мира спортивных ставок с минимальными рисками. Не упускайте свой шанс и начните своё приключение в БК Лига Ставок с нашим фрибетом. Заберите свой фрибет сейчас же и стартуйте к выигрышам!
Nellyownyv
April 17, 2024 at 7:31 amРады сообщить о выпуске эксклюзивного промокода для БК Лига Ставок! Этот специальный промокод открывает доступ к особым бонусам и повышает ваш игровой процесс, делая его ещё более выгодным и волнующим. [url=https://ligastavok-promocode.ru/]Ligastavok промокод[/url] для активации сегодня! С его использованием, игроки получают доступ к дополнительным бонусам на ставки, ускоренной процедуре регистрации и эксклюзивным акциям. Воспользуйтесь этой возможностью стать частью элитного клуба пользователей БК Лига Ставок и максимизируйте свои выигрыши с нашим промокодом. Используйте код прямо сейчас и заберите свои бонусы еще до первой ставки!
Nellygpcms
April 17, 2024 at 8:09 amПредставляем доступности эксклюзивного промокода для БК Лига Ставок! Этот специальный промокод предоставляет доступ к эксклюзивным привилегиям и усиливает ваш опыт ставок, делая его ещё более выгодным и захватывающим. [url=https://ligastavok-promocode.ru/]Промокод на лига ставок[/url] для активации сегодня! С его использованием, игроки получают доступ к дополнительным бонусам на ставки, ускоренной процедуре регистрации и специальным предложениям. Не упустите шанс стать частью привилегированной группы пользователей БК Лига Ставок и максимизируйте свои выигрыши с нашим промокодом. Активируйте промокод прямо сейчас и начните выигрывать еще до первой ставки!
Nellyfrekh
April 17, 2024 at 8:58 amРады сообщить о выпуске эксклюзивного промокода для БК Лига Ставок! Этот специальный промокод открывает доступ к эксклюзивным привилегиям и усиливает ваш игровой процесс, делая его ещё более выгодным и захватывающим. [url=https://ligastavok-promocode.ru/]Промокоды на лига ставок[/url] для активации сегодня! С данным промокодом, игроки получают доступ к дополнительным бонусам на ставки, ускоренной процедуре регистрации и эксклюзивным акциям. Воспользуйтесь этой возможностью стать частью элитного клуба пользователей БК Лига Ставок и увеличьте свои шансы на успех с нашим промокодом. Используйте код уже сегодня и заберите свои бонусы еще до первой ставки!
Nellywaxqt
April 17, 2024 at 9:28 amПредставляем выпуске эксклюзивного промокода для БК Лига Ставок! Этот специальный промокод открывает доступ к эксклюзивным привилегиям и повышает ваш игровой процесс, делая его ещё более выгодным и захватывающим. [url=https://ligastavok-promocode.ru/]Промокоды лига ставок[/url] после ввода кода! С данным промокодом, игроки получают непосредственный доступ к бонусным средствам, привилегиям при регистрации и эксклюзивным акциям. Не упустите шанс стать частью привилегированной группы пользователей БК Лига Ставок и увеличьте свои шансы на успех с нашим промокодом. Активируйте промокод уже сегодня и начните выигрывать еще до первой ставки!
Manrealudo
April 17, 2024 at 9:53 amСамый ожидаемый футбольный поединок сезона – Манчестер Сити против Реала Мадрид! Азарт, волнение, и адреналин ждут нас на поле. Но этот день может быть еще более захватывающим благодаря промокоду PLAYDAY от 1win! Ставьте на своего фаворита с уверенностью и удвойте свои шансы на победу! Не упустите шанс – забирайте [url=https://www.pinterest.com/pin/916693699143021366/]Манчестер Сити Реал Мадрид бонус код за регистрацию[/url] уже сегодня!
Manrealirr
April 17, 2024 at 10:31 amСамый ожидаемый футбольный поединок сезона – Манчестер Сити против Реала Мадрид! Страсть, нервы, и адреналин ждут нас на поле. Но этот день может быть еще более увлекательным благодаря промокоду PLAYDAY от 1win! Ставьте на своего любимца с уверенностью и растите свои шансы на победу! Не упустите шанс – забирайте [url=https://www.pinterest.com/pin/916693699143021366/]Манчестер Сити Реал Мадрид бонус[/url] уже сегодня!
Manreallbo
April 17, 2024 at 11:20 amСамый ожидаемый футбольный поединок сезона – Манчестер Сити против Реала Мадрид! Энтузиазм, волнение, и невероятные эмоции ждут нас на поле. Но этот день может быть еще более захватывающим благодаря промокоду PLAYDAY от 1win! Ставьте на своего фаворита с уверенностью и повысьте свои шансы на победу! Не упустите шанс – забирайте [url=https://www.pinterest.com/pin/916693699143021366/]Манчестер Сити Реал Мадрид бонус 500[/url] уже сегодня!
Manrealdwx
April 17, 2024 at 11:50 amСамый ожидаемый футбольный поединок сезона – Манчестер Сити против Реала Мадрид! Азарт, нервы, и адреналин ждут нас на поле. Но этот день может быть еще более увлекательным благодаря промокоду PLAYDAY от 1win! Ставьте на своего фаворита с уверенностью и повысьте свои шансы на победу! Не упустите шанс – забирайте [url=https://www.pinterest.com/pin/916693699143021366/]Манчестер Сити Реал Мадрид промокод[/url] уже сегодня!
BAARSjtum
April 17, 2024 at 12:31 pmБитва гигантов на поле – Бавария против Арсенала! Волнение, напряжение и невообразимые моменты ждут нас в этом схватке. Но почему бы не добавить еще больше эмоций с помощью промокода PLAYDAY от 1win? Сделайте ставку на победную команду и увеличьте свои выигрышные возможности! Не упустите этот момент – активируйте [url=https://www.pinterest.com/pin/916693699143021391]Бавария Арсенал бонус[/url] уже сегодня и радуйтесь игры в полную силу!
BAARSfdeh
April 17, 2024 at 1:10 pmБитва гигантов на поле – Бавария против Арсенала! Эмоции, возбуждение и невероятные моменты ждут нас в этом схватке. Но почему бы не добавить еще больше адреналина с помощью промокода PLAYDAY от 1win? Сделайте ставку на победителя и увеличьте свои шансы на победу! Не упустите этот возможность – воспользуйтесь [url=https://www.pinterest.com/pin/916693699143021391]Бавария Арсенал бонус 500[/url] уже сегодня и наслаждайтесь игры в полную силу!
winpromonn
April 17, 2024 at 2:03 pmВстречайте игру на новом уровне с промокодом PLAYDAY от 1win! Независимо от того, на какую событие вы ставите – будь то спортивное противостояние – этот промокод приносит вам дополнительные шансы для победы. Увеличьте свои выигрышные шансы, активируя промокод PLAYDAY при размещении ставок. Не упустите шанс на увлекательный опыт и бонусные выигрыши – активируйте [url=https://www.pinterest.com/pin/916693699143021313/]1win бонус на первый депозит[/url] прямо сейчас на 1win!
winpromxdw
April 17, 2024 at 2:42 pmВстречайте матч на высшем уровне с промокодом PLAYDAY от 1win! Независимо от того, на какую событие вы делаете ставку – будь то футбольная битва – этот промокод приносит вам экстра-шансы для победы. Расти свои шансы на успех, активируя промокод PLAYDAY при размещении ставок. Не упустите шанс на потрясающие впечатления и дополнительные выигрыши – используйте [url=https://www.pinterest.com/pin/916693699143021313/]1win промокод на деньги при пополнении[/url] прямо сейчас на 1win!
winprompua
April 17, 2024 at 3:30 pmВстречайте спортивное событие на высшем уровне с промокодом PLAYDAY от 1win! Независимо от того, на какую событие вы делаете ставку – будь то спортивное противостояние – этот промокод приносит вам экстра-шансы для победы. Расти свои шансы на успех, используя промокод PLAYDAY при размещении ставок. Не упустите возможность на потрясающие впечатления и дополнительные выигрыши – воспользуйтесь [url=https://www.pinterest.com/pin/916693699143021313/]1win бонусы конторы[/url] прямо сейчас на 1win!
winpromttd
April 17, 2024 at 4:00 pmВстречайте матч на новом уровне с промокодом PLAYDAY от 1win! Независимо от того, на какую событие вы делаете ставку – будь то спортивное противостояние – этот промокод приносит вам дополнительные шансы для победы. Расти свои шансы на успех, активируя промокод PLAYDAY при размещении ставок. Не упустите шанс на потрясающие впечатления и бонусные выигрыши – используйте [url=https://www.pinterest.com/pin/916693699143021313/]промокод при регистрации 1win[/url] прямо сейчас на 1win!
Zolktroks
April 17, 2024 at 6:42 pmdoes tamsulosin cause high blood pressure
Brokerorly
April 17, 2024 at 7:25 pmВ настоящее время наши дни могут содержать внезапные издержки и финансовые вызовы, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]деньги в кредит[/url] вам помогут без избыточной бюрократии. В своей роли представителя я стремлюсь распространить эту информацию, чтобы помочь тем, кто столкнулся с временными трудностями. Наша платформа обеспечивает прозрачные условия и быструю обработку заявок, чтобы каждый мог разрешить свои финансовые вопросы быстро и без лишних хлопот.
Brokerufgx
April 17, 2024 at 8:53 pmВ настоящее время наши дни могут содержать неожиданные расходы и финансовые вызовы, и в такие моменты каждый ищет надежную поддержку. На сайте [url=https://brokers-group.ru/]получить деньги в долг в Минске[/url] вам помогут без избыточной бюрократии. В своей роли агента я стремлюсь распространить эту информацию, чтобы помочь людям в сложной ситуации. Наша система обеспечивает прозрачные условия и быструю обработку заявок, чтобы каждый мог решить свои денежные проблемы быстро и без лишних хлопот.
Brokerustp
April 17, 2024 at 9:24 pmВ настоящее время наши дни могут содержать внезапные издержки и экономические трудности, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]получить деньги в долг[/url] вам помогут без лишних сложностей. В своей роли агента я стремлюсь распространить эту информацию, чтобы помочь тем, кто столкнулся с временными трудностями. Наша платформа обеспечивает прозрачные условия и быструю обработку заявок, чтобы каждый мог разрешить свои финансовые вопросы оперативно и без лишних затрат времени.
Dengizpkjr
April 18, 2024 at 12:54 amВ наше время многие из нас сталкиваются с непредвиденными расходами и экономическими вызовами, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]взять деньги в долг[/url] вам помогут быстро и удобно. В качестве официального представителя Брокерс Групп, я рад делиться этой информацией, чтобы помочь людям, кто нуждается в финансовой поддержке. Наша система гарантирует честные условия и быструю обработку заявок, чтобы каждый мог решить свои финансовые вопросы быстро и эффективно.
Dengizivnu
April 18, 2024 at 1:33 amВ наше время многие из нас сталкиваются с внезапными затратами и денежными трудностями, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]dengizaimy.ru[/url] вам помогут быстро и удобно. В качестве представителя Брокерс Групп, я рад делиться этой информацией, чтобы помочь каждому, кто нуждается в финансовой поддержке. Наша платформа гарантирует прозрачные условия и быструю обработку заявок, чтобы каждый мог решить свои финансовые вопросы быстро и эффективно.
Dengizjcfa
April 18, 2024 at 2:22 amВ наше время многие из нас сталкиваются с непредвиденными расходами и денежными трудностями, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]быстрый займ[/url] вам помогут быстро и удобно. В качестве представителя Брокерс Групп, я рад делиться этой важной информацией, чтобы помочь каждому, кто нуждается в финансовой поддержке. Наша платформа гарантирует честные условия и оперативное рассмотрение запросов, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
Dengizwjht
April 18, 2024 at 2:54 amВ наше время многие из нас сталкиваются с непредвиденными расходами и экономическими вызовами, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]dengizaimy.ru[/url] вам помогут с минимальными усилиями. В качестве представителя Брокерс Групп, я с удовольствием делиться этой важной информацией, чтобы помочь каждому, кто столкнулся с финансовыми трудностями. Наша система гарантирует честные условия и оперативное рассмотрение запросов, чтобы каждый мог разрешить свои денежные проблемы с минимальными временными затратами.
Moneybellj
April 18, 2024 at 6:19 amВ сегодняшнем обществе финансовые сложности не редкость, и каждый из нас может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]займы онлайн[/url] помогут преодолеть эти трудности, предоставляя простой и удобный способ займа. Мы осознаём, что времени на решение проблемы не хватает, поэтому мы обрабатываем заявки оперативно и эффективно. В качестве представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предложив ясные и выгодные условия займа. Доверьтесь нашей компании, и мы решим ваши финансовые проблемы.
Moneybeldy
April 18, 2024 at 6:59 amВ настоящей эпохе финансовые сложности не редкость, и каждый из нас может оказаться в ситуации, когда потребуется дополнительная финансовая помощь. На сайте [url=https://moneybel.ru/]moneybel[/url] помогут решить эти проблемы, предоставляя простой и удобный способ займа. Мы осознаём, что время играет ключевую роль, поэтому мы обрабатываем заявки оперативно и эффективно. В роли представителя Манибел, мы нацелены на то, чтобы помочь каждому клиенту преодолеть свои денежные трудности, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нам, и мы поможем вам в решении ваших финансовых проблем.
Moneybelgp
April 18, 2024 at 8:02 amВ настоящей эпохе финансовые сложности не редкость, и каждый человек может оказаться в ситуации, когда потребуется дополнительная финансовая помощь. На сайте [url=https://moneybel.ru/]деньги в долг[/url] помогут преодолеть эти трудности, предоставляя простой и лёгкий метод займа. Мы понимаем, что время играет ключевую роль, поэтому мы обрабатываем заявки оперативно и эффективно. В качестве представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нам, и мы поможем вам в решении ваших финансовых проблем.
Hottest Hairstyles
April 18, 2024 at 8:17 amThanks for this glorious article. Yet another thing to mention is that almost all digital cameras can come equipped with a zoom lens so that more or less of the scene to get included through ‘zooming’ in and out. These changes in target length usually are reflected while in the viewfinder and on significant display screen on the back of this camera.
Moneybelzy
April 18, 2024 at 8:32 amВ сегодняшнем обществе финансовые трудности не редкость, и каждый из нас может оказаться в ситуации, когда потребуется дополнительная финансовая помощь. На сайте [url=https://moneybel.ru/]взять деньги в долг[/url] помогут преодолеть эти трудности, предоставляя простой и лёгкий метод получения кредита. Мы осознаём, что время играет ключевую роль, поэтому наш процесс обработки заявок максимально быстр и эффективен. В роли представителя Манибел, мы нацелены на то, чтобы помочь каждому клиенту преодолеть свои денежные трудности, предложив ясные и выгодные условия займа. Доверьтесь нашей компании, и мы поможем вам в решении ваших финансовых проблем.
CnnyIrrer
April 18, 2024 at 9:02 amtizanidine 2mg side effects
lentaibfo
April 18, 2024 at 11:36 amBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]bellenty.by[/url] помогут консультанты интернет-магазина. Благодаря нашему опыту и профессионализму мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам стандартам качества и эффективности. Мы изготавливаем индивидуальные решения, учитывая особые потребности наших клиентов. Независимо от величины, материала или технических характеристик, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentafail
April 18, 2024 at 12:17 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]стоимость конвейерной ленты в Минске[/url] помогут консультанты интернет-магазина. Благодаря нашему опыту и компетентности мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам стандартам качества и эффективности. Мы изготавливаем индивидуальные решения, учитывая особые потребности наших клиентов. Независимо от величины, материала или технических характеристик, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentamico
April 18, 2024 at 1:06 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]цена транспортерной ленты на Bellenty.by[/url] помогут консультанты магазина. Благодаря нашему опыту и компетентности мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам стандартам качества и эффективности. Мы разрабатываем индивидуальные решения, учитывая уникальные потребности наших заказчиков. Независимо от величины, материала или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentadlbh
April 18, 2024 at 1:36 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]купить конвейерную ленту[/url] помогут консультанты магазина. Благодаря нашему опыту и профессионализму мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам стандартам качества и эффективности. Мы разрабатываем индивидуальные решения, учитывая особые потребности наших заказчиков. Независимо от размера, состава или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
AyybScuse
April 18, 2024 at 4:48 pminteractions for voltaren gel
Hairstyles
April 18, 2024 at 9:37 pmIts like you read my mind! You appear to know so much about this, like you wrote the book in it or something. I think that you could do with some pics to drive the message home a little bit, but other than that, this is excellent blog. A fantastic read. I will definitely be back.
Bbbfanect
April 19, 2024 at 4:29 amvenlafaxine dosage for depression
AyybScuse
April 19, 2024 at 4:54 amvoltaren+gel+1%
Bbbfanect
April 19, 2024 at 5:53 amtapering off venlafaxine
CnnyIrrer
April 19, 2024 at 7:56 amtizanidine how long in system
Zoljtroks
April 19, 2024 at 10:21 pmzyprexa metabolism
CndyIrrer
April 20, 2024 at 12:24 pmdrug interaction tramadol and zofran
Moneybelcf
April 20, 2024 at 12:27 pmВ сегодняшнем обществе финансовые сложности встречаются часто, и каждый человек может оказаться в ситуации, когда потребуется дополнительная финансовая помощь. На сайте [url=https://moneybel.ru/]деньги в долг в Минске[/url] помогут преодолеть эти трудности, предоставляя простой и удобный способ займа. Мы осознаём, что время играет ключевую роль, поэтому наш процесс обработки заявок максимально быстр и эффективен. В качестве представителя Манибел, мы нацелены на то, чтобы помочь каждому клиенту преодолеть свои денежные трудности, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нам, и мы решим ваши финансовые проблемы.
Moneybellg
April 20, 2024 at 1:05 pmВ настоящей эпохе финансовые трудности встречаются часто, и каждый человек может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]деньги в долг в Минске[/url] помогут преодолеть эти трудности, принося простой и удобный способ получения кредита. Мы понимаем, что времени на решение проблемы не хватает, поэтому наш процесс обработки заявок максимально быстр и эффективен. В качестве представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нашей компании, и мы поможем вам в решении ваших финансовых проблем.
Moneybelkk
April 20, 2024 at 1:53 pmВ сегодняшнем обществе финансовые трудности не редкость, и каждый человек может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]получить деньги[/url] помогут преодолеть эти трудности, принося простой и удобный способ займа. Мы осознаём, что времени на решение проблемы не хватает, поэтому мы обрабатываем заявки оперативно и эффективно. В качестве представителя Манибел, мы нацелены на то, чтобы помочь каждому клиенту преодолеть свои денежные трудности, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нам, и мы поможем вам в решении ваших финансовых проблем.
Moneybelpp
April 20, 2024 at 2:24 pmВ настоящей эпохе финансовые трудности встречаются часто, и каждый из нас может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]деньги в долг срочно[/url] помогут решить эти проблемы, принося простой и удобный способ займа. Мы понимаем, что времени на решение проблемы не хватает, поэтому мы обрабатываем заявки оперативно и эффективно. В роли представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нам, и мы решим ваши финансовые проблемы.
Zaimhyhsp
April 20, 2024 at 3:50 pmВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]деньги в долг в Минске[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
Zaimceqiy
April 20, 2024 at 4:29 pmВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]взять деньги в кредит[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
Zaimwvqjv
April 20, 2024 at 5:17 pmВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]ЗаймМинск[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
Zaimworzj
April 20, 2024 at 5:48 pmВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]взять деньги в долг[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
lentarhak
April 20, 2024 at 7:15 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]цены на конвейерные ленты[/url] помогут консультанты магазина. Благодаря нашему опыту и профессионализму мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам качества и эффективности. Мы изготавливаем индивидуальные решения, учитывая особые потребности наших заказчиков. Независимо от размера, материала или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentabzsn
April 20, 2024 at 7:54 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]цена конвейерной ленты[/url] помогут консультанты интернет-магазина. Благодаря нашему опыту и компетентности мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам качества и эффективности. Мы разрабатываем индивидуальные решения, учитывая уникальные потребности наших заказчиков. Независимо от величины, состава или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
Larrymom
April 20, 2024 at 8:36 pmPBN sites
We will create a system of private blog network sites!
Benefits of our PBN network:
We carry out everything SO THAT Google does not understand THAT this is A privately-owned blog network!!!
1- We obtain domains from different registrars
2- The main site is hosted on a virtual private server (Virtual Private Server is high-speed hosting)
3- Other sites are on separate hostings
4- We attribute a separate Google ID to each site with confirmation in Search Console.
5- We design websites on WP, we don’t use plugins with assistance from which malware penetrate and through which pages on your websites are created.
6- We do not reiterate templates and utilise only individual text and pictures
We refrain from work with website design; the client, if wanted, can then edit the websites to suit his wishes
lentahbkv
April 20, 2024 at 8:43 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]цены на ленты конвейерные[/url] помогут консультанты магазина. Благодаря нашему опыту и профессионализму мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам стандартам качества и эффективности. Мы изготавливаем индивидуальные решения, учитывая уникальные потребности наших клиентов. Независимо от величины, материала или технических характеристик, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentafscx
April 20, 2024 at 9:14 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]заказать транспортерную ленту на Bellenty.by[/url] помогут консультанты магазина. Благодаря нашему опыту и компетентности мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам стандартам качества и эффективности. Мы изготавливаем индивидуальные решения, учитывая особые потребности наших клиентов. Независимо от величины, состава или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
Brokerkhqh
April 20, 2024 at 10:40 pmВ настоящее время наши дни могут содержать внезапные издержки и финансовые вызовы, и в такие моменты каждый ищет надежную поддержку. На сайте [url=https://brokers-group.ru/]деньги в долг[/url] вам помогут без лишних сложностей. В своей роли агента я стремлюсь распространить эту информацию, чтобы помочь людям в сложной ситуации. Наша платформа обеспечивает прозрачные условия и быструю обработку заявок, чтобы каждый мог решить свои денежные проблемы быстро и без лишних хлопот.
Brokerdgai
April 20, 2024 at 11:19 pmВ настоящее время наши дни могут содержать внезапные издержки и экономические трудности, и в такие моменты каждый ищет надежную поддержку. На сайте [url=https://brokers-group.ru/]получить деньги в долг[/url] вам помогут без избыточной бюрократии. В своей роли агента я стремлюсь поделиться этими сведениями, чтобы помочь тем, кто столкнулся с временными трудностями. Наша платформа обеспечивает прозрачные условия и быструю обработку заявок, чтобы каждый мог разрешить свои финансовые вопросы оперативно и без лишних затрат времени.
Brokerqlkr
April 21, 2024 at 12:08 amВ настоящее время наши дни могут содержать неожиданные расходы и экономические трудности, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]получить деньги в долг[/url] вам помогут без избыточной бюрократии. В своей роли представителя я стремлюсь поделиться этими сведениями, чтобы помочь тем, кто столкнулся с временными трудностями. Наша система обеспечивает честные условия и быструю обработку заявок, чтобы каждый мог разрешить свои финансовые вопросы быстро и без лишних хлопот.
Brokerquam
April 21, 2024 at 12:40 amВ настоящее время наши дни могут содержать внезапные издержки и экономические трудности, и в такие моменты каждый ищет надежную поддержку. На сайте [url=https://brokers-group.ru/]деньги в долг[/url] вам помогут без лишних сложностей. В своей роли агента я стремлюсь распространить эту информацию, чтобы помочь тем, кто столкнулся с временными трудностями. Наша платформа обеспечивает честные условия и быструю обработку заявок, чтобы каждый мог решить свои денежные проблемы оперативно и без лишних затрат времени.
Dengizgnny
April 21, 2024 at 2:06 amВ наше время многие из нас сталкиваются с внезапными затратами и экономическими вызовами, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]взять деньги в долг в Минске[/url] вам помогут с минимальными усилиями. В качестве представителя Брокерс Групп, я с удовольствием делиться этой информацией, чтобы помочь людям, кто нуждается в финансовой поддержке. Наша система гарантирует прозрачные условия и оперативное рассмотрение запросов, чтобы каждый мог решить свои финансовые вопросы быстро и эффективно.
Dengizxhql
April 21, 2024 at 2:46 amВ наше время многие из нас сталкиваются с непредвиденными расходами и денежными трудностями, и в такие моменты важно иметь возможность взять кредит. На сайте [url=https://dengizaimy.ru/]деньги в долг[/url] вам помогут с минимальными усилиями. В качестве представителя Брокерс Групп, я рад делиться этой важной информацией, чтобы помочь каждому, кто столкнулся с финансовыми трудностями. Наша система гарантирует честные условия и быструю обработку заявок, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
Dengiznylv
April 21, 2024 at 3:34 amВ наше время многие из нас сталкиваются с непредвиденными расходами и денежными трудностями, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]получить займ в Минске[/url] вам помогут быстро и удобно. В качестве официального представителя Брокерс Групп, я с удовольствием делиться этой важной информацией, чтобы помочь каждому, кто нуждается в финансовой поддержке. Наша платформа гарантирует прозрачные условия и оперативное рассмотрение запросов, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
Dengizbdkt
April 21, 2024 at 4:05 amВ наше время многие из нас сталкиваются с внезапными затратами и денежными трудностями, и в такие моменты важно иметь возможность взять кредит. На сайте [url=https://dengizaimy.ru/]оформить займ[/url] вам помогут с минимальными усилиями. В качестве официального представителя Брокерс Групп, я рад делиться этой важной информацией, чтобы помочь людям, кто столкнулся с финансовыми трудностями. Наша система гарантирует прозрачные условия и быструю обработку заявок, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
Dengizerly
April 21, 2024 at 10:28 amВ наше время многие из нас сталкиваются с внезапными затратами и экономическими вызовами, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]займ в РБ[/url] вам помогут быстро и удобно. В качестве официального представителя Брокерс Групп, я с удовольствием делиться этой важной информацией, чтобы помочь каждому, кто столкнулся с финансовыми трудностями. Наша платформа гарантирует честные условия и быструю обработку заявок, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
Dengizhnks
April 21, 2024 at 11:09 amВ наше время многие из нас сталкиваются с внезапными затратами и денежными трудностями, и в такие моменты важно иметь возможность взять кредит. На сайте [url=https://dengizaimy.ru/]деньги в долг[/url] вам помогут быстро и удобно. В качестве представителя Брокерс Групп, я с удовольствием делиться этой важной информацией, чтобы помочь каждому, кто столкнулся с финансовыми трудностями. Наша платформа гарантирует прозрачные условия и быструю обработку заявок, чтобы каждый мог разрешить свои денежные проблемы с минимальными временными затратами.
Dengizqgmy
April 21, 2024 at 12:00 pmВ наше время многие из нас сталкиваются с внезапными затратами и денежными трудностями, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]взять деньги в долг в Минске[/url] вам помогут с минимальными усилиями. В качестве официального представителя Брокерс Групп, я рад делиться этой информацией, чтобы помочь людям, кто столкнулся с финансовыми трудностями. Наша система гарантирует прозрачные условия и быструю обработку заявок, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
CndyIrrer
April 21, 2024 at 12:08 pmzofran actions
Dengizszdq
April 21, 2024 at 12:32 pmВ наше время многие из нас сталкиваются с внезапными затратами и денежными трудностями, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]взять деньги[/url] вам помогут быстро и удобно. В качестве официального представителя Брокерс Групп, я с удовольствием делиться этой информацией, чтобы помочь людям, кто столкнулся с финансовыми трудностями. Наша платформа гарантирует прозрачные условия и быструю обработку заявок, чтобы каждый мог разрешить свои денежные проблемы с минимальными временными затратами.
Moneybelkc
April 21, 2024 at 2:47 pmВ сегодняшнем обществе финансовые сложности встречаются часто, и каждый из нас может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]займ на карту без отказа[/url] помогут решить эти проблемы, предоставляя простой и удобный способ получения кредита. Мы понимаем, что времени на решение проблемы не хватает, поэтому мы обрабатываем заявки оперативно и эффективно. В качестве представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предложив ясные и выгодные условия займа. Доверьтесь нам, и мы решим ваши финансовые проблемы.
Moneybelog
April 21, 2024 at 3:28 pmВ сегодняшнем обществе финансовые трудности не редкость, и каждый из нас может оказаться в ситуации, когда потребуется дополнительная финансовая помощь. На сайте [url=https://moneybel.ru/]moneybel.ru[/url] помогут решить эти проблемы, предоставляя простой и удобный способ получения кредита. Мы осознаём, что время играет ключевую роль, поэтому мы обрабатываем заявки оперативно и эффективно. В роли представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предложив ясные и выгодные условия займа. Доверьтесь нашей компании, и мы решим ваши финансовые проблемы.
Moneybelqy
April 21, 2024 at 4:19 pmВ сегодняшнем обществе финансовые трудности встречаются часто, и каждый из нас может оказаться в ситуации, когда потребуется дополнительная финансовая помощь. На сайте [url=https://moneybel.ru/]moneybel.ru[/url] помогут преодолеть эти трудности, предоставляя простой и лёгкий метод займа. Мы осознаём, что время играет ключевую роль, поэтому мы обрабатываем заявки оперативно и эффективно. В качестве представителя Манибел, мы нацелены на то, чтобы помочь каждому клиенту преодолеть свои денежные трудности, предложив ясные и выгодные условия займа. Доверьтесь нашей компании, и мы поможем вам в решении ваших финансовых проблем.
Moneybelbb
April 21, 2024 at 4:50 pmВ сегодняшнем обществе финансовые трудности встречаются часто, и каждый человек может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]займы онлайн[/url] помогут преодолеть эти трудности, предоставляя простой и лёгкий метод займа. Мы осознаём, что времени на решение проблемы не хватает, поэтому мы обрабатываем заявки оперативно и эффективно. В роли представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нам, и мы поможем вам в решении ваших финансовых проблем.
Zaimrvewl
April 21, 2024 at 7:10 pmВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]займы онлайн на карту[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
AmmhScuse
April 21, 2024 at 7:48 pmezetimibe (zetia)
Zaimcebxq
April 21, 2024 at 7:50 pmВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]деньги в долг в Минске[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
Zaimkmscn
April 21, 2024 at 8:40 pmВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]взять деньги в долг в Минске[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
Zaimamxye
April 21, 2024 at 9:11 pmВ настоящей экономической обстановке, где неожиданные финансовые обязательства могут возникнуть в любое время, поиск надежного и удобного источника финансовой помощи становится все более критическим. Предложение [url=https://zaim-minsk.ru/]взять деньги[/url] предлагает возможность рассчитывать на быстрое и беззаботное получение необходимых средств без лишних трудностей. Мы ценим ваше время и обеспечиваем оперативную обработку всех запросов. В качестве вашего финансового партнера, наша главная задача состоит в том, чтобы помочь вам преодолеть текущие финансовые препятствия, предоставляя ясные и выгодные условия кредитования. Доверьтесь нам в вашем финансовом путешествии, и мы обеспечим вас надежной поддержкой на каждом этапе.
lentaadha
April 21, 2024 at 10:37 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]транспортерные ленты на Bellenty.by[/url] помогут консультанты магазина. Благодаря нашему опыту и компетентности мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам качества и эффективности. Мы изготавливаем индивидуальные решения, учитывая уникальные потребности наших заказчиков. Независимо от величины, состава или технических характеристик, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentanzre
April 21, 2024 at 11:17 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]оформить конвейерную ленту[/url] помогут консультанты интернет-магазина. Благодаря нашему практике и профессионализму мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам качества и эффективности. Мы разрабатываем индивидуальные решения, учитывая уникальные потребности наших заказчиков. Независимо от величины, материала или технических характеристик, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentarqsi
April 22, 2024 at 12:07 amBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]купить ленту транспортерную в Минске[/url] помогут консультанты интернет-магазина. Благодаря нашему опыту и профессионализму мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам качества и эффективности. Мы производим индивидуальные решения, учитывая уникальные потребности наших клиентов. Независимо от величины, материала или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentahkoa
April 22, 2024 at 12:39 amBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]заказать конвейерную ленту в Минске[/url] помогут консультанты магазина. Благодаря нашему опыту и компетентности мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам качества и эффективности. Мы изготавливаем индивидуальные решения, учитывая уникальные потребности наших заказчиков. Независимо от размера, состава или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
Zoljtroks
April 22, 2024 at 1:47 amzyprexa drowsiness
Brokerngth
April 22, 2024 at 2:05 amВ настоящее время наши дни могут содержать неожиданные расходы и финансовые вызовы, и в такие моменты каждый ищет надежную поддержку. На сайте [url=https://brokers-group.ru/]Брокерс Групп[/url] вам помогут без избыточной бюрократии. В своей роли агента я стремлюсь распространить эту информацию, чтобы помочь тем, кто столкнулся с временными трудностями. Наша система обеспечивает прозрачные условия и быструю обработку заявок, чтобы каждый мог решить свои денежные проблемы быстро и без лишних хлопот.
Brokerwpda
April 22, 2024 at 2:45 amВ настоящее время наши дни могут содержать внезапные издержки и экономические трудности, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]взять деньги в долг[/url] вам помогут без лишних сложностей. В своей роли агента я стремлюсь поделиться этими сведениями, чтобы помочь тем, кто столкнулся с временными трудностями. Наша система обеспечивает честные условия и быструю обработку заявок, чтобы каждый мог разрешить свои финансовые вопросы быстро и без лишних хлопот.
Brokerniqq
April 22, 2024 at 3:35 amВ настоящее время наши дни могут содержать внезапные издержки и финансовые вызовы, и в такие моменты каждый ищет надежную поддержку. На сайте [url=https://brokers-group.ru/]займ денег[/url] вам помогут без избыточной бюрократии. В своей роли агента я стремлюсь распространить эту информацию, чтобы помочь людям в сложной ситуации. Наша платформа обеспечивает честные условия и эффективное рассмотрение запросов, чтобы каждый мог разрешить свои финансовые вопросы быстро и без лишних хлопот.
Brokerweqg
April 22, 2024 at 4:07 amВ настоящее время наши дни могут содержать неожиданные расходы и финансовые вызовы, и в такие моменты каждый ищет надежную поддержку. На сайте [url=https://brokers-group.ru/]оформить займ в Минске[/url] вам помогут без избыточной бюрократии. В своей роли агента я стремлюсь поделиться этими сведениями, чтобы помочь тем, кто столкнулся с временными трудностями. Наша платформа обеспечивает честные условия и быструю обработку заявок, чтобы каждый мог разрешить свои финансовые вопросы оперативно и без лишних затрат времени.
Haircuts
April 22, 2024 at 4:50 amI learned more new things on this fat reduction issue. A single issue is that good nutrition is highly vital whenever dieting. A massive reduction in bad foods, sugary meals, fried foods, sugary foods, red meat, and white colored flour products might be necessary. Keeping wastes bloodsuckers, and toxins may prevent desired goals for fat-loss. While certain drugs in the short term solve the challenge, the unpleasant side effects usually are not worth it, they usually never give more than a short-lived solution. It is just a known fact that 95 of dietary fads fail. Many thanks for sharing your ideas on this web site.
Dengizaeqk
April 22, 2024 at 5:32 amВ наше время многие из нас сталкиваются с непредвиденными расходами и денежными трудностями, и в такие моменты важно иметь возможность взять кредит. На сайте [url=https://dengizaimy.ru/]деньги в долг[/url] вам помогут быстро и удобно. В качестве официального представителя Брокерс Групп, я рад делиться этой важной информацией, чтобы помочь людям, кто столкнулся с финансовыми трудностями. Наша система гарантирует прозрачные условия и оперативное рассмотрение запросов, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
Dengizxgux
April 22, 2024 at 6:12 amВ наше время многие из нас сталкиваются с внезапными затратами и денежными трудностями, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]ДеньгиЗаймы[/url] вам помогут быстро и удобно. В качестве представителя Брокерс Групп, я рад делиться этой информацией, чтобы помочь каждому, кто нуждается в финансовой поддержке. Наша платформа гарантирует честные условия и быструю обработку заявок, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
Dengizzckk
April 22, 2024 at 7:02 amВ наше время многие из нас сталкиваются с внезапными затратами и денежными трудностями, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]получить займ[/url] вам помогут с минимальными усилиями. В качестве официального представителя Брокерс Групп, я рад делиться этой важной информацией, чтобы помочь каждому, кто нуждается в финансовой поддержке. Наша платформа гарантирует честные условия и оперативное рассмотрение запросов, чтобы каждый мог разрешить свои денежные проблемы с минимальными временными затратами.
Bmooanect
April 22, 2024 at 7:34 amconsecuencias de tomar wellbutrin
Dengizvaea
April 22, 2024 at 7:34 amВ наше время многие из нас сталкиваются с внезапными затратами и экономическими вызовами, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]деньги в долг[/url] вам помогут быстро и удобно. В качестве официального представителя Брокерс Групп, я с удовольствием делиться этой информацией, чтобы помочь каждому, кто нуждается в финансовой поддержке. Наша система гарантирует честные условия и оперативное рассмотрение запросов, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
AmmhScuse
April 22, 2024 at 7:50 amzetia medication
Bmooanect
April 22, 2024 at 8:58 amwellbutrin work for anxiety
lentaaazs
April 22, 2024 at 2:43 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]стоимость транспортерной ленты в Минске[/url] помогут консультанты интернет-магазина. Благодаря нашему опыту и компетентности мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам стандартам качества и эффективности. Мы производим индивидуальные решения, учитывая уникальные потребности наших клиентов. Независимо от величины, материала или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentaincd
April 22, 2024 at 3:23 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]заказать транспортерную ленту[/url] помогут консультанты интернет-магазина. Благодаря нашему опыту и профессионализму мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам стандартам качества и эффективности. Мы изготавливаем индивидуальные решения, учитывая особые потребности наших клиентов. Независимо от величины, материала или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
CndyIrrer
April 22, 2024 at 3:34 pmside effects of zofran in children
lentaogdf
April 22, 2024 at 4:13 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]заказать транспортерную ленту[/url] помогут консультанты магазина. Благодаря нашему опыту и компетентности мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам качества и эффективности. Мы производим индивидуальные решения, учитывая особые потребности наших заказчиков. Независимо от размера, состава или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentapyqf
April 22, 2024 at 4:44 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]оформить ленты конвейерные на Bellenty.by[/url] помогут консультанты магазина. Благодаря нашему опыту и профессионализму мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам качества и эффективности. Мы производим индивидуальные решения, учитывая уникальные потребности наших клиентов. Независимо от размера, состава или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentamzjy
April 22, 2024 at 6:10 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]транспортерные ленты на Bellenty[/url] помогут консультанты интернет-магазина. Благодаря нашему практике и профессионализму мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам стандартам качества и эффективности. Мы изготавливаем индивидуальные решения, учитывая особые потребности наших клиентов. Независимо от величины, материала или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentatyji
April 22, 2024 at 6:50 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]оформить транспортерную ленту на Bellenty.by[/url] помогут консультанты магазина. Благодаря нашему практике и компетентности мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам качества и эффективности. Мы изготавливаем индивидуальные решения, учитывая уникальные потребности наших заказчиков. Независимо от размера, состава или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentajywi
April 22, 2024 at 7:41 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]стоимость ленты конвейерной в Минске[/url] помогут консультанты магазина. Благодаря нашему опыту и компетентности мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам качества и эффективности. Мы изготавливаем индивидуальные решения, учитывая особые потребности наших заказчиков. Независимо от размера, состава или технических характеристик, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
lentaywbz
April 22, 2024 at 8:13 pmBellenty – ваш надёжный союзник в области конвейерных решений. Для вас [url=http://bellenty.by/]стоимость конвейерных лент в Минске[/url] помогут консультанты магазина. Благодаря нашему опыту и компетентности мы гарантируем, что каждая лента, предлагаемая нами, соответствует высочайшим стандартам качества и эффективности. Мы производим индивидуальные решения, учитывая особые потребности наших заказчиков. Независимо от величины, материала или спецификаций, Bellenty обеспечивает идеальное решение для вашего конвейерного оборудования, даря вашему бизнесу надёжность и эффективность.
Brokercxaz
April 22, 2024 at 10:41 pmВ настоящее время наши дни могут содержать неожиданные расходы и экономические трудности, и в такие моменты каждый ищет надежную поддержку. На сайте [url=https://brokers-group.ru/]деньги в долг[/url] вам помогут без лишних сложностей. В своей роли агента я стремлюсь поделиться этими сведениями, чтобы помочь тем, кто столкнулся с временными трудностями. Наша система обеспечивает честные условия и эффективное рассмотрение запросов, чтобы каждый мог решить свои денежные проблемы оперативно и без лишних затрат времени.
Brokerwpjh
April 22, 2024 at 11:22 pmВ настоящее время наши дни могут содержать внезапные издержки и экономические трудности, и в такие моменты каждый ищет надежную поддержку. На сайте [url=https://brokers-group.ru/]деньги в долг[/url] вам помогут без лишних сложностей. В своей роли агента я стремлюсь распространить эту информацию, чтобы помочь тем, кто столкнулся с временными трудностями. Наша система обеспечивает прозрачные условия и эффективное рассмотрение запросов, чтобы каждый мог решить свои денежные проблемы оперативно и без лишних затрат времени.
Brokerelnp
April 23, 2024 at 12:13 amВ настоящее время наши дни могут содержать неожиданные расходы и экономические трудности, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]получить деньги в долг[/url] вам помогут без лишних сложностей. В своей роли агента я стремлюсь поделиться этими сведениями, чтобы помочь людям в сложной ситуации. Наша система обеспечивает честные условия и эффективное рассмотрение запросов, чтобы каждый мог разрешить свои финансовые вопросы быстро и без лишних хлопот.
Brokerywth
April 23, 2024 at 12:45 amВ настоящее время наши дни могут содержать неожиданные расходы и финансовые вызовы, и в такие моменты каждый ищет помощь. На сайте [url=https://brokers-group.ru/]Брокерс Групп[/url] вам помогут без лишних сложностей. В своей роли представителя я стремлюсь поделиться этими сведениями, чтобы помочь людям в сложной ситуации. Наша платформа обеспечивает прозрачные условия и быструю обработку заявок, чтобы каждый мог разрешить свои финансовые вопросы оперативно и без лишних затрат времени.
Dengizapdq
April 23, 2024 at 2:10 amВ наше время многие из нас сталкиваются с непредвиденными расходами и экономическими вызовами, и в такие моменты важно иметь возможность взять кредит. На сайте [url=https://dengizaimy.ru/]взять деньги в кредит[/url] вам помогут быстро и удобно. В качестве представителя Брокерс Групп, я с удовольствием делиться этой информацией, чтобы помочь каждому, кто нуждается в финансовой поддержке. Наша система гарантирует честные условия и оперативное рассмотрение запросов, чтобы каждый мог решить свои финансовые вопросы с минимальными временными затратами.
Dengizxsbk
April 23, 2024 at 2:51 amВ наше время многие из нас сталкиваются с непредвиденными расходами и экономическими вызовами, и в такие моменты важно иметь возможность взять кредит. На сайте [url=https://dengizaimy.ru/]взять деньги в долг[/url] вам помогут с минимальными усилиями. В качестве официального представителя Брокерс Групп, я с удовольствием делиться этой информацией, чтобы помочь каждому, кто столкнулся с финансовыми трудностями. Наша платформа гарантирует честные условия и оперативное рассмотрение запросов, чтобы каждый мог разрешить свои денежные проблемы быстро и эффективно.
Dengiznkwz
April 23, 2024 at 3:41 amВ наше время многие из нас сталкиваются с внезапными затратами и экономическими вызовами, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]займ на карту без отказа[/url] вам помогут с минимальными усилиями. В качестве представителя Брокерс Групп, я рад делиться этой информацией, чтобы помочь каждому, кто столкнулся с финансовыми трудностями. Наша система гарантирует прозрачные условия и оперативное рассмотрение запросов, чтобы каждый мог решить свои финансовые вопросы с минимальными временными затратами.
Dengizvdby
April 23, 2024 at 4:13 amВ наше время многие из нас сталкиваются с непредвиденными расходами и экономическими вызовами, и в такие моменты важно иметь доступ к дополнительным средствам. На сайте [url=https://dengizaimy.ru/]взять деньги в долг[/url] вам помогут с минимальными усилиями. В качестве официального представителя Брокерс Групп, я с удовольствием делиться этой информацией, чтобы помочь каждому, кто нуждается в финансовой поддержке. Наша платформа гарантирует честные условия и быструю обработку заявок, чтобы каждый мог разрешить свои денежные проблемы с минимальными временными затратами.
Moneybelho
April 23, 2024 at 5:38 amВ настоящей эпохе финансовые трудности не редкость, и каждый человек может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]МаниБел[/url] помогут решить эти проблемы, предоставляя простой и лёгкий метод получения кредита. Мы понимаем, что времени на решение проблемы не хватает, поэтому наш процесс обработки заявок максимально быстр и эффективен. В роли представителя Манибел, наша цель – помочь каждому клиенту преодолеть финансовые затруднения, предложив ясные и выгодные условия займа. Доверьтесь нам, и мы решим ваши финансовые проблемы.
Moneybelkg
April 23, 2024 at 6:18 amВ настоящей эпохе финансовые сложности не редкость, и каждый из нас может оказаться в ситуации, когда потребуется дополнительная финансовая помощь. На сайте [url=https://moneybel.ru/]взять деньги в кредит[/url] помогут решить эти проблемы, принося простой и удобный способ займа. Мы понимаем, что времени на решение проблемы не хватает, поэтому мы обрабатываем заявки оперативно и эффективно. В качестве представителя Манибел, мы нацелены на то, чтобы помочь каждому клиенту преодолеть свои денежные трудности, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нам, и мы поможем вам в решении ваших финансовых проблем.
Moneybelhs
April 23, 2024 at 7:08 amВ настоящей эпохе финансовые сложности встречаются часто, и каждый из нас может оказаться в ситуации, когда необходима дополнительная финансовая поддержка. На сайте [url=https://moneybel.ru/]деньги в долг в Минске срочно[/url] помогут решить эти проблемы, принося простой и удобный способ получения кредита. Мы осознаём, что время играет ключевую роль, поэтому мы обрабатываем заявки оперативно и эффективно. В качестве представителя Манибел, мы нацелены на то, чтобы помочь каждому клиенту преодолеть свои денежные трудности, предоставив прозрачные и выгодные условия кредитования. Доверьтесь нашей компании, и мы решим ваши финансовые проблемы.
Olimpxethx
April 23, 2024 at 7:32 amЗапускайте свежую версию приложения БК Олимп для Android и измените свои стандарты в мире ставок! [url=https://apk-olimp.ru/]Приложение БК Олимп[/url] доступно для всех. С современным дизайном и быстрой загрузкой данных, доступ к различным спортивным событиям становится игрой. Управление аккаунтом и навигация по приложению теперь так удобны, что каждая ставка превращается в радость. Скачайте последнюю версию прямо сейчас и начните добиваться успеха с БК Олимп, где каждый шаг может привести к большой победе!
Olimpweuar
April 23, 2024 at 8:13 amЗапускайте новую версию приложения БК Олимп для Android и измените свои стандарты в мире ставок! [url=https://apk-olimp.ru/]Приложение Олимп бет[/url] прямо сейчас. С современным дизайном и ускоренной загрузкой данных, доступ к различным спортивным событиям становится легкостью. Ведение счета и навигация по приложению теперь так удобны, что каждая ставка превращается в радость. Установите последнюю версию без задержек и начните выигрывать с БК Олимп, где каждый шаг может привести к значимому триумфу!
Olimpebfib
April 23, 2024 at 9:04 amЗапускайте свежую версию приложения БК Олимп для Android и переопределите свои стандарты в мире ставок! [url=https://apk-olimp.ru/]БК Олимп для андроид[/url] доступно для всех. С современным дизайном и ускоренной загрузкой данных, доступ к различным спортивным событиям становится легкостью. Управление аккаунтом и перемещение по разделам теперь так удобны, что каждая ставка превращается в удовольствие. Скачайте последнюю версию без задержек и начните добиваться успеха с БК Олимп, где каждый шаг может привести к значимому триумфу!
Olimppkksd
April 23, 2024 at 9:36 amЗапускайте новую версию приложения БК Олимп для Android и переопределите свои стандарты в мире ставок! [url=https://apk-olimp.ru/]Скачать Олимп бет на андроид[/url] уже сейчас. С передовым дизайном и ускоренной загрузкой данных, доступ к многочисленным спортивным событиям становится легкостью. Управление аккаунтом и перемещение по разделам теперь так удобны, что каждая ставка превращается в удовольствие. Скачайте последнюю версию без задержек и начните выигрывать с БК Олимп, где каждый шаг может привести к большой победе!
Olimpfyymm
April 23, 2024 at 3:40 pmОткройте для себя перспективы ставок с обновленным приложением БК Олимп для Android! Обновленный дизайн и быстрая загрузка обеспечивают легкий доступ к многообразию спортивных событий. [url=https://app-olimb.ru/]Скачать БК Олимп для андроид[/url] для всех. Упрощенное управление счетом и легкость использования делают ставки удовольствием. Установите это приложение немедленно и повышайте свои шансы в ставках с БК Олимп, где каждое событие предоставляет шанс на победу!
Olimpwoers
April 23, 2024 at 4:21 pmОткройте для себя перспективы ставок с обновленным приложением БК Олимп для Android! Обновленный дизайн и быстрая загрузка обеспечивают легкий доступ к широкому ассортименту спортивных событий. [url=https://app-olimb.ru/]Скачать Olimp[/url] для всех. Упрощенное управление счетом и легкость использования делают ставки приятным занятием. Установите это обновление сейчас же и повышайте свои шансы в ставках с БК Олимп, где каждое событие предоставляет шанс на успех!
Olimplqmjt
April 23, 2024 at 5:12 pmОткройте для себя перспективы ставок с обновленным приложением БК Олимп для Android! Переосмысленный дизайн и ускоренная обработка данных обеспечивают легкий доступ к широкому ассортименту спортивных событий. [url=https://app-olimb.ru/]Олимп на андроид[/url] для всех. Простота ведения финансов и легкость использования делают ставки удовольствием. Загрузите это приложение сейчас же и выходите на новый уровень в ставках с БК Олимп, где каждое событие предоставляет шанс на победу!
Olimpmxlff
April 23, 2024 at 5:44 pmОткройте для себя новый горизонт ставок с обновленным приложением БК Олимп для Android! Переосмысленный дизайн и ускоренная обработка данных обеспечивают легкий доступ к многообразию спортивных событий. [url=https://app-olimb.ru/]Скачать БК Олимп[/url] для вас. Упрощенное управление счетом и интуитивная навигация делают ставки приятным занятием. Установите это приложение немедленно и повышайте свои шансы в ставках с БК Олимп, где каждое событие предоставляет шанс на победу!
Olimpbiyxt
April 24, 2024 at 6:38 amЗапускайте новую версию приложения БК Олимп для Android и переопределите свои стандарты в мире ставок! [url=https://apk-olimp.ru/]Олимп на андроид[/url] уже сегодня. С современным дизайном и ускоренной загрузкой данных, доступ к различным спортивным событиям становится игрой. Управление аккаунтом и перемещение по разделам теперь настолько просты, что каждая ставка превращается в радость. Скачайте последнюю версию без задержек и начните добиваться успеха с БК Олимп, где каждый шаг может привести к значимому триумфу!
Olimpaoyah
April 24, 2024 at 7:19 amЗапускайте новую версию приложения БК Олимп для Android и переопределите свои стандарты в мире ставок! [url=https://apk-olimp.ru/]Olimp bet на андроид[/url] уже сегодня. С современным дизайном и быстрой загрузкой данных, доступ к различным спортивным событиям становится игрой. Ведение счета и перемещение по разделам теперь так удобны, что каждая ставка превращается в радость. Установите последнюю версию прямо сейчас и начните выигрывать с БК Олимп, где каждый шаг может привести к большой победе!
Olimpgsbed
April 24, 2024 at 8:11 amЗапускайте свежую версию приложения БК Олимп для Android и переопределите свои стандарты в мире ставок! [url=https://apk-olimp.ru/]Скачать Olimp на андроид[/url] доступно для всех. С передовым дизайном и быстрой загрузкой данных, доступ к различным спортивным событиям становится легкостью. Управление аккаунтом и навигация по приложению теперь так удобны, что каждая ставка превращается в удовольствие. Установите последнюю версию без задержек и начните добиваться успеха с БК Олимп, где каждый шаг может привести к значимому триумфу!
Olimpmqtrv
April 24, 2024 at 8:44 amЗапускайте новую версию приложения БК Олимп для Android и измените свои стандарты в мире ставок! [url=https://apk-olimp.ru/]БК Олимп для андроид[/url] уже сейчас. С современным дизайном и быстрой загрузкой данных, доступ к различным спортивным событиям становится легкостью. Управление аккаунтом и навигация по приложению теперь так удобны, что каждая ставка превращается в удовольствие. Скачайте последнюю версию прямо сейчас и начните выигрывать с БК Олимп, где каждый шаг может привести к большой победе!
Olimpcaohg
April 24, 2024 at 9:39 amОткройте для себя новый горизонт ставок с обновленным приложением БК Олимп для Android! Обновленный дизайн и ускоренная обработка данных обеспечивают легкий доступ к широкому ассортименту спортивных событий. [url=https://app-olimb.ru/]Скачать на андроид букмекерскую Олимп[/url] для каждого. Простота ведения финансов и легкость использования делают ставки удовольствием. Установите это обновление сейчас же и повышайте свои шансы в ставках с БК Олимп, где каждое событие предоставляет шанс на победу!
Olimpkwazn
April 24, 2024 at 10:21 amОткройте для себя перспективы ставок с обновленным приложением БК Олимп для Android! Переосмысленный дизайн и быстрая загрузка обеспечивают легкий доступ к широкому ассортименту спортивных событий. [url=https://app-olimb.ru/]Скачать Олимп для андроид[/url] для вас. Упрощенное управление счетом и интуитивная навигация делают ставки приятным занятием. Установите это обновление сейчас же и выходите на новый уровень в ставках с БК Олимп, где каждое событие предоставляет шанс на успех!
Hottest Hairstyles
April 24, 2024 at 10:38 amGreat paintings! That is the type of information that are meant to be shared around the internet. Disgrace on the seek engines for not positioning this put up upper! Come on over and discuss with my website . Thank you =)
Olimpvgqoj
April 24, 2024 at 11:12 amОткройте для себя перспективы ставок с обновленным приложением БК Олимп для Android! Переосмысленный дизайн и ускоренная обработка данных обеспечивают легкий доступ к многообразию спортивных событий. [url=https://app-olimb.ru/]Скачать Olimp[/url] для всех. Простота ведения финансов и интуитивная навигация делают ставки удовольствием. Установите это обновление сейчас же и повышайте свои шансы в ставках с БК Олимп, где каждое событие предоставляет шанс на победу!
Olimppijfx
April 24, 2024 at 11:45 amОткройте для себя перспективы ставок с обновленным приложением БК Олимп для Android! Переосмысленный дизайн и быстрая загрузка обеспечивают легкий доступ к многообразию спортивных событий. [url=https://app-olimb.ru/]Olimp для андроид[/url] для всех. Простота ведения финансов и интуитивная навигация делают ставки приятным занятием. Загрузите это приложение сейчас же и повышайте свои шансы в ставках с БК Олимп, где каждое событие предоставляет шанс на успех!
LazaroBeece
April 24, 2024 at 12:48 pmЗакажите SEO продвижение сайта https://seo116.ru/ в Яндекс и Google под ключ в Москве и по всей России от экспертов. Увеличение трафика, рост клиентов, онлайн поддержка. Комплексное продвижение сайтов с гарантией.
w-495.ru
April 24, 2024 at 8:11 pmчас с психологом w-495.ru
order zithromax over the counter
April 25, 2024 at 10:58 am[url=http://azithromycinps.online/]azithromycin 500 mg tablet price[/url]
buy prednisone without prescription
April 25, 2024 at 12:04 pm[url=http://predniso.online/]where can i buy prednisone without prescription[/url]
Scottret
April 25, 2024 at 1:24 pmUnderstanding COSC Accreditation and Its Importance in Watchmaking
COSC Certification and its Demanding Criteria
Controle Officiel Suisse des Chronometres, or the Official Swiss Chronometer Testing Agency, is the authorized Switzerland testing agency that attests to the precision and accuracy of wristwatches. COSC validation is a sign of quality craftsmanship and trustworthiness in timekeeping. Not all timepiece brands seek COSC accreditation, such as Hublot, which instead sticks to its own stringent criteria with movements like the UNICO calibre, achieving similar precision.
The Art of Precision Chronometry
The core system of a mechanized timepiece involves the mainspring, which delivers power as it unwinds. This system, however, can be prone to environmental elements that may impact its accuracy. COSC-validated movements undergo demanding testing—over 15 days in various conditions (5 positions, three temperatures)—to ensure their durability and dependability. The tests assess:
Typical daily rate accuracy between -4 and +6 seconds.
Mean variation, peak variation rates, and effects of thermal changes.
Why COSC Accreditation Is Important
For timepiece enthusiasts and connoisseurs, a COSC-accredited watch isn’t just a piece of tech but a proof to enduring quality and precision. It symbolizes a watch that:
Presents excellent reliability and accuracy.
Ensures assurance of quality across the whole construction of the watch.
Is likely to maintain its value more efficiently, making it a smart investment.
Well-known Chronometer Manufacturers
Several famous brands prioritize COSC accreditation for their timepieces, including Rolex, Omega, Breitling, and Longines, among others. Longines, for instance, presents collections like the Record and Spirit, which feature COSC-certified movements equipped with advanced materials like silicone equilibrium suspensions to enhance resilience and efficiency.
Historical Context and the Evolution of Chronometers
The idea of the timepiece dates back to the requirement for precise chronometry for navigation at sea, emphasized by John Harrison’s work in the 18th century. Since the formal establishment of COSC in 1973, the certification has become a yardstick for evaluating the precision of luxury timepieces, continuing a legacy of superiority in horology.
Conclusion
Owning a COSC-certified watch is more than an visual selection; it’s a dedication to excellence and accuracy. For those appreciating accuracy above all, the COSC accreditation offers peace of thoughts, guaranteeing that each certified timepiece will function dependably under various circumstances. Whether for individual contentment or as an investment decision, COSC-validated watches distinguish themselves in the world of watchmaking, carrying on a legacy of precise chronometry.
Scottret
April 25, 2024 at 6:49 pm網上賭場
Vittyspdrj
April 26, 2024 at 8:33 amПриветствуем вас в мир ставок с БК Лига Ставок! Процесс регистрации и входа в личный кабинет созданы для максимального удобства и безопасности каждого пользователя. [url=https://ligastavok-login.ru/]Зарегистрироваться в букмекерской конторе лига ставок[/url] – легко и быстро, и это открывает доступ к широкому спектру функций: от ставок на спорт до участия в акциях и получения бонусов.
Начните с нескольких легких шагов для регистрации: заполните обязательные поля формы, подтвердите свою электронную почту и номер телефона, и вы готовы к старту! После регистрации, используйте ваш новый аккаунт с помощью полученных данных – и весь мир ставок БК Лига Ставок окажется у вас под рукой.
Ваш личный кабинет – это командный пункт вашими ставками, где вы можете легко пополнять счет, участвовать в играх, просматривать историю транзакций, а также получать эксклюзивные предложения и уведомления о предстоящих событиях. Не теряйте времени – присоединяйтесь к БК Лига Ставок уже сегодня и погрузитесь в мир ставок с комфортом и уверенностью!
Georgebit
April 26, 2024 at 1:12 pmvovan casino
Levaxkewb
April 26, 2024 at 3:05 pmАнонсируем выход обновленной мобильного приложения БК Лига Ставок для Android! Этот шаг полностью преобразует ваш пользовательский опыт, делая его ещё удобнее и динамичным. [url=https://ligastavok-android.ru/]Лига ставок на андроид[/url] уже сегодня! В обновлении вы найдете прямой доступ к огромному ассортименту спортивных мероприятий из любой точки с помощью вашего устройства. Усовершенствованное управление профилем, инновационная концепция дизайна для удобной навигации и увеличенная скорость работы приложения – всё это создано для вас. Станьте частью воодушевленных пользователей и наслаждайтесь возможностями от ставок в любой точке планеты и в любое время. Установите обновление приложения БК Лига Ставок уже сегодня и переходите к новому этапу игры!
CharlesEdibe
April 26, 2024 at 4:46 pmКакие радиаторы выбрать для отопления https://propest.ru/kak-vybrat-radiator-dlya-doma.html частного загородного дома – каменного или деревянного: разновидности и классы, правила выбора, цены, сравнения.
Scottret
April 26, 2024 at 5:06 pmSon Dönemin En Fazla Gözde Kumarhane Platformu: Casibom
Bahis oyunlarını sevenlerin artık duymuş olduğu Casibom, son dönemde adından çoğunlukla söz ettiren bir bahis ve oyun platformu haline geldi. Ülkemizdeki en mükemmel casino platformlardan biri olarak tanınan Casibom’un haftalık bazda olarak değişen açılış adresi, sektörde oldukça yenilikçi olmasına rağmen emin ve kar getiren bir platform olarak öne çıkıyor.
Casibom, muadillerini geride bırakarak köklü kumarhane platformların üstünlük sağlamayı başarıyor. Bu alanda uzun soluklu olmak önemli olsa da, oyunculardan iletişimde bulunmak ve onlara ulaşmak da aynı derecede önemlidir. Bu noktada, Casibom’un her saat servis veren gerçek zamanlı destek ekibi ile kolayca iletişime geçilebilir olması büyük bir artı sağlıyor.
Süratle artan katılımcı kitlesi ile dikkat çekici olan Casibom’un gerisindeki başarı faktörleri arasında, yalnızca casino ve canlı olarak casino oyunlarıyla sınırlı olmayan geniş bir hizmet yelpazesi bulunuyor. Atletizm bahislerinde sunduğu geniş seçenekler ve yüksek oranlar, oyuncuları cezbetmeyi başarmayı sürdürüyor.
Ayrıca, hem sporcular bahisleri hem de kumarhane oyunları oyuncularına yönlendirilen sunulan yüksek yüzdeli avantajlı promosyonlar da ilgi çekiyor. Bu nedenle, Casibom hızla piyasada iyi bir pazarlama başarısı elde ediyor ve büyük bir oyuncuların kitlesi kazanıyor.
Casibom’un kazandıran ödülleri ve popülerliği ile birlikte, siteye üyelik nasıl sağlanır sorusuna da atıfta bulunmak gereklidir. Casibom’a taşınabilir cihazlarınızdan, bilgisayarlarınızdan veya tabletlerinizden internet tarayıcı üzerinden rahatça erişilebilir. Ayrıca, platformun mobil uyumlu olması da büyük bir artı sağlıyor, çünkü artık hemen hemen herkesin bir cep telefonu var ve bu akıllı telefonlar üzerinden kolayca giriş sağlanabiliyor.
Mobil tabletlerinizle bile yolda canlı olarak tahminler alabilir ve müsabakaları gerçek zamanlı olarak izleyebilirsiniz. Ayrıca, Casibom’un mobil uyumlu olması, memleketimizde kumarhane ve oyun gibi yerlerin kanuni olarak kapatılmasıyla birlikte bu tür platformlara girişin önemli bir yolunu oluşturuyor.
Casibom’un emin bir casino sitesi olması da önemli bir fayda sağlıyor. Ruhsatlı bir platform olan Casibom, duraksız bir şekilde eğlence ve kar elde etme imkanı sağlar.
Casibom’a kullanıcı olmak da oldukça kolaydır. Herhangi bir belge gereksinimi olmadan ve ücret ödemeden siteye rahatça kullanıcı olabilirsiniz. Ayrıca, site üzerinde para yatırma ve çekme işlemleri için de birçok farklı yöntem bulunmaktadır ve herhangi bir kesim ücreti isteseniz de alınmaz.
Ancak, Casibom’un güncel giriş adresini izlemek de gereklidir. Çünkü gerçek zamanlı iddia ve oyun web siteleri moda olduğu için sahte web siteleri ve dolandırıcılar da görünmektedir. Bu nedenle, Casibom’un sosyal medya hesaplarını ve güncel giriş adresini düzenli aralıklarla kontrol etmek elzemdir.
Sonuç, Casibom hem güvenilir hem de kar getiren bir casino platformu olarak dikkat çekici. yüksek promosyonları, kapsamlı oyun alternatifleri ve kullanıcı dostu taşınabilir uygulaması ile Casibom, oyun sevenler için ideal bir platform getiriyor.
Lianaitbkc
April 26, 2024 at 9:44 pmВашему вниманию предлагается эксклюзивное предложение от БК Лига Ставок – фрибет для новых игроков! Это бесплатная ставка позволяет вам испытать удачу без риска потери собственных средств, открывая новые горизонты для получения призов с первых же шагов в мире ставок. [url=https://ligastavok-freebet.ru/]Лига ставок фрибет при регистрации[/url] для всех новичков после регистрации! С фрибетом от БК Лига Ставок, вы имеете возможность ознакомиться с платформой без каких-либо финансовых обязательств. Это идеальный старт для тех, кто хочет стать частью мира спортивных ставок с минимальными рисками. Не упускайте свой шанс и начните своё приключение в БК Лига Ставок с нашим фрибетом. Заберите свой фрибет уже сегодня и стартуйте к выигрышам!
Nellywqqvb
April 27, 2024 at 3:46 amПредставляем выпуске эксклюзивного промокода для БК Лига Ставок! Этот специальный промокод предоставляет доступ к эксклюзивным привилегиям и повышает ваш игровой процесс, делая его более прибыльным и волнующим. [url=https://ligastavok-promocode.ru/]Лига ставок промокод[/url] для активации сегодня! С данным промокодом, игроки получают доступ к дополнительным бонусам на ставки, привилегиям при регистрации и эксклюзивным акциям. Воспользуйтесь этой возможностью стать частью элитного клуба пользователей БК Лига Ставок и максимизируйте свои выигрыши с нашим промокодом. Активируйте промокод прямо сейчас и начните выигрывать еще до первой ставки!